Your cart is currently empty!
How to make a custom Response in Laravel
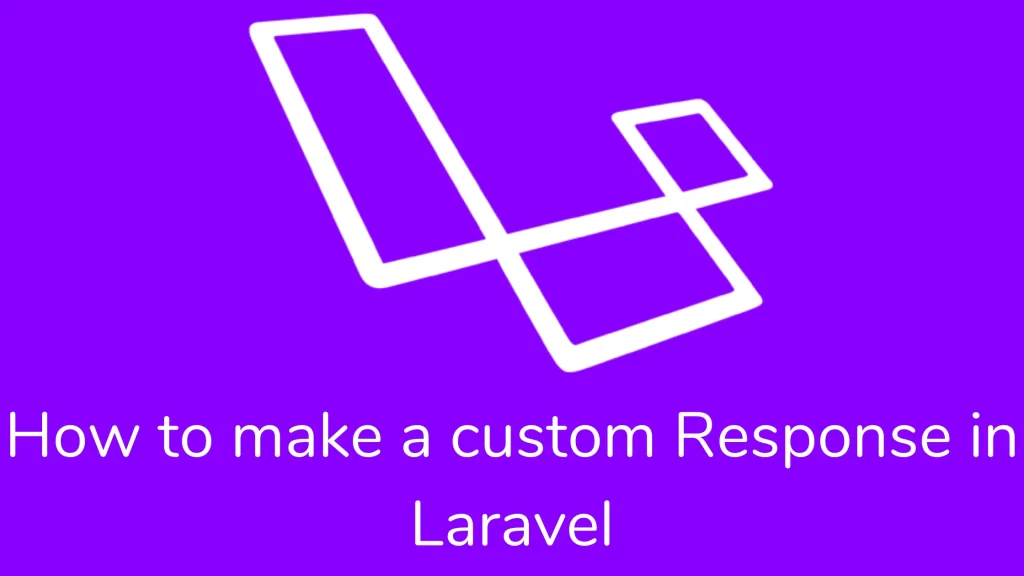
Hi guys,
Today we learned to make a custom response in Laravel and handle HTTP headers. We can change parameters from URLs inside the model.
Creating Custom response in laravel:-
All routes and controllers need to return a response to set back to the user’s browser. Laravel provides different ways to return responses. The framework will automatically convert the string into a full HTTP response.
Route::get('/', function(){
return 'Hello World';
})
You may also return arrays. The framework will automatically convert an array into JSON format.
Route::get('/', function(){
return [1,2,4,5,3];
});
Attaching header to response:
Returning a full Response instance allows you to customize the response’s HTTP status code and headers. You want to assign a single HTTP header to the response so that we can use the header() method like the below:
Route::get('home', function () {
return response('Hello World', 200)
->header('Content-Type', 'text/plain');
});
You want to assign more headers to the response. We can use the withHeaders() method with an array like below:
Route::get('home', function(){
return response('hello world')->withHeaders([
'Content-Type' => 'text/plain',
'X-Header-One' => 'Header One',
'X-Header-Two' => 'Header Two',
]);
})
Cache controller middleware:
‘cache.headers’ middleware may set the cache-control header for a group of routes. An MD5 hash of the response content will automatically be set as the ETag identifier.
Route::middleware('cache.headers:public;max_age=2628000;etag')->group(function () {
Route::get('privacy', function () {
// …
});
Route::get('terms', function () {
// ...
});
});
You can easily add a cookie to the response using the cookie() method, and you can pass arguments the same as the setcookie() method in the PHP language.
return response($content)
->header('Content-Type', $type)
->cookie('name', 'value', $minutes);
Cookie and Encryption:
All cookies are encrypted and signed so that they can’t be changed or read by the client. You want to disable encryption for cookies generated by the application. You can add new value inside $except the property of EncryptCookies middleware.
/**
The names of the cookies that should not be encrypted.
*
@var array
*/
protected $except = [
'cookie_name',
];
Redirects:-
You need to redirect one view to another view. You can use a global redirect() helper function.
Route::get('dashboard', function () {
return redirect('home/dashboard');
});
When a submitted form has an invalid value, you may send it back to the form using the global back helper function.
Route::post('user/profile', function() {
return back()->withInput();
});
Redirecting To Named Routes:
You want to use a named route in the controller. You can use the route() method of the Redirector instance.
return redirect()->route('login');
If you want to pass a parameter to the URL using a named route. You can pass the second argument to the route method:
return redirect()->route('profile',['id'=>1]);
Populating parameters via models:
You may pass the model itself. It will extract id from the model.
return redirect()->route('profile',[$user]);
If you want to pass a parameter to the URL using a named route. You can pass the second argument to the route method:
public function getRouteKey()
{
return $this->slug;
}
Redirect to controller Actions:
You can also redirect to controller actions using the action() method. Just pass the controller name with the action name. If the activity requires arguments, you can give an array as a second parameter.
return redirect()->action('HomeController@index');
return redirect()->action(
'UserController@profile', ['id' => 1]
);
Redirecting To external domains:
The away() method is to redirect an external domain.
return redirect()->away('http://www.google.com');
Redirecting with flashed session data:
You want to display a success message after redirecting to a new URL. You can set session messages using the ‘with()’ method.
Route::post('user/profile', function(){
return redirect('dashboard')->with('status', 'Password Saved');
});
On the view side, you can write below code:
@if (session('status'))
<div class="alert alert-success">
{{ session('status') }}
</div>
@endif
Other Response Types:-
The response() helper function is to generate other response types.
View:
If you want to control HTTP status and headers and return a view as response content, use the view() method. HTTP status and the HTTP header is optional.
return response()->view('hello', $data,200)->header('Content-Type', $type);
JSON Responses:
All Type of API sends data into JSON format. Laravel has a JSON method that automatically changes Content-Type to application/JSON and converts an array to JSON format.
return response()->json([
'name' => 'Abigail',
'state' => 'CA'
]);
You can make a JSONP response using the JSON() method with the withCallback() method.
return response()->json([
'name' => 'Abigail',
'state' => 'CA'
])->withCallback($request->input('callback'));
File Downloads:
The download() method forcefully downloads the file from a path. You can pass three arguments to the download() method first argument is a path of the file, the second argument is the filename which will display while downloading, and the third argument is an array of headers.
return response()->download($pathToFile);
return response()->download($pathToFile, $name, $headers);
return response()->download($pathToFile)->deleteFileAfterSend();
Streamed download:
The streamDownload method dynamically generates a response in a downloadable format without storing content on disk.
return response()->streamDownload(function () {
echo GitHub::api('repo')
->contents()
->readme('laravel', 'laravel')['contents'];
}, 'laravel-readme.md');
File Responses:
The file method returns display content on the user’s browser, which is an image or pdf. The file method accepts two arguments: the first argument is the file path, and the second argument is an array of headers.
return response()->file($pathToFile);
return response()->file($pathToFile, $headers);
Response Macros:-
You can create a response that you can reuse in your routes and controllers. For Example, we can write a macro function inside the boot method.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Response;
use Illuminate\Support\ServiceProvider;
class ResponseMacroServiceProvider extends ServiceProvider
{
/**
* Register the application's response macros.
*
* @return void
*/
public function boot()
{
Response::macro('caps', function ($value) {
return Response::make(strtoupper($value));
});
}
}
The macro() function has two arguments: a name as its first argument and closure as its second argument. Macro’s closure will execute when calling the macro name from ResponseFactory and response helper.
return response()->caps('foo');
I am pretty sure this article (How to make Custom Response in laravel) it helps you a better understanding of the response in Laravel. I have followed this link for this article. If you have any doubts, then comment below then I will reply as per as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,
Thanks designed for sharing such a good thinking, piece of writing is nice, thats why i have read it completely Lanna Alvin Stoat