Your cart is currently empty!
What is the use of service providers for laravel
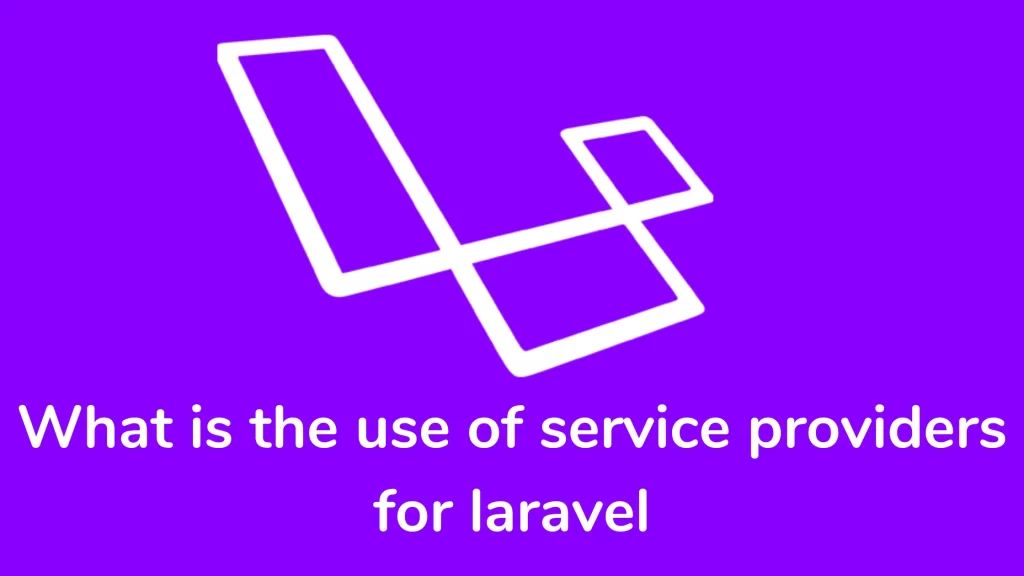
Hi guys,
In this article, We are studying service providers for laravel. It is the central place of all laravel application bootstrapping. You can be bootstrapping custom classes and laravel core classes, which contain registering service container bindings, event listeners, middleware, and custom routes.
You can see providers inside the config/app.php file. It contains the Providers array. You can specify your service providers and already contain a set of laravel core service providers and Provider loads on a specific route. You will learn how to write and register your service provider in the laravel application.
Create a Service Provider
All Service Provider extends the Illuminate\Support\ServiceProvider class. Run the below command to make your service provider.
php artisan make:provider InstallServiceProvider
It contains a register and boot method. In the register method, you can specify only bind things into the service container. You should not register any event listeners or routes.
Register method:
You should not register any event listeners, routes, or functionality within the register method of a service container. Instead, only bind things in the container. You can access the $app property, which provides access to the service container.
<?php
namespace App\Providers;
use App\Services\Riak\Connection;
use Illuminate\Support\ServiceProvider;
class RiakServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
$this->app->singleton(Connection::class, function ($app) {
return new Connection(config('riak'));
});
}
}
Bindings and Singletons Properties:
You want to register many service providers in a simple binding using bindings and singletons properties. It will automatically check these properties and register their bindings.
<?php
namespace App\Providers;
use App\Contracts\DowntimeNotifier;
use App\Contracts\ServerProvider;
use App\Services\DigitalOceanServerProvider;
use App\Services\PingdomDowntimeNotifier;
use App\Services\ServerToolsProvider;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* All of the container bindings that should registered.
*
* @var array
*/
public $bindings = [
ServerProvider::class => DigitalOceanServerProvider::class,
];
/**
* All of the container singletons that should be registered.
*
* @var array
*/
public $singletons = [
DowntimeNotifier::class => PingdomDowntimeNotifier::class,
ServerProvider::class => ServerToolsProvider::class,
];
}
Boot Method:
This function is invoked after the successful registration of all other service providers. You can access all registered service providers.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\View;
use Illuminate\Support\ServiceProvider;
class ComposerServiceProvider extends ServiceProvider
{
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
View::composer('view', function () {
//
});
}
}
Registering Providers
The config\app.php contains all service providers. All Laravel core service providers are listed in the array by default against the Provider’s array.
'providers' => [
// Other Service Providers
App\Providers\ComposerServiceProvider::class,
],
Deferred Providers:
You have the option to delay registration until a registered binding is required. Such a provider will improve the performance of your application because it hasn’t loaded on every request from the filesystem. Laravel now compiles and stores a list of deferred service providers, including their service provider classes. If you want to implement a deferred provider, you should implement \Illuminate\Contracts\Support\Deferrable Provider interface and define provides a method. The provides method should return the service container bindings registered by the providers.
<?php
namespace App\Providers;
use App\Services\Riak\Connection;
use Illuminate\Contracts\Support\DeferrableProvider;
use Illuminate\Support\ServiceProvider;
class RiakServiceProvider extends ServiceProvider implements DeferrableProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
$this->app->singleton(Connection::class, function ($app) {
return new Connection($app['config']['riak']);
});
}
/**
* Get the services provided by the providers.
*
* @return array
*/
public function provides()
{
return [Connection::class];
}
}
Service providers are crucial for Laravel application bootstrapping, enabling registration of service container bindings, event listeners, middleware, and custom routes. They can be created using the php artisan make:provider InstallServiceProvider command and their bindings can be checked and registered using bindings and singletons properties.
You can check developer tools on the Supertools website. Please give me feedback on this website.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers