Your cart is currently empty!
Top 6 Easy Steps to Implement Events in Laravel
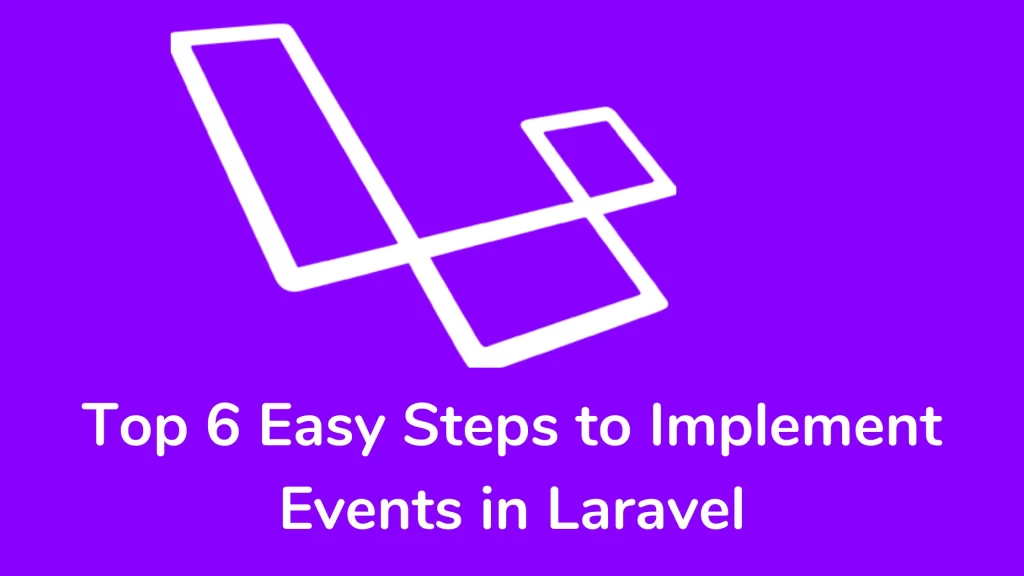
Hi guys,
Events follow an observer pattern that allows you to subscribe and listen to events inside your application. In the directory structure, All Event classes are stored in the app/Events directory, and we need a listener for them, which is stored in the app/Listeners directory.
Follow the below 6 steps to implement events in laravel
Generate events and listeners:-
If you want to add listeners and events to your EventServiceProvider file, use the following event:generate command
php artisan event:generate
You can generate events and listeners through the following command.
php artisan make:event OrderPlaced
php artisan make:listener SendOrderPlacedNotification --event=OrderPlaced
Also, we can manually add events and listeners in the EventServiceProvider file. Events should be registered via EventServiceProvider $listen array.
use App\Events\PodcastProcessed;
use App\Listeners\SendPodcastNotification;
use Illuminate\Support\Facades\Event;
/**
* Register any other events for your application.
*/
public function boot(): void
{
Event::listen(
OrderPlaced::class,
[OrderPlacedNotification::class, 'handle']
);
Event::listen(function (OrderPlaced $event) {
// ...
});
}
You can wrap the queue of the event class in the EventServiceProvider file using the following code.
use App\Events\PodcastProcessed;
use function Illuminate\Events\queueable;
use Illuminate\Support\Facades\Event;
/**
* Register any other events for your application.
*/
public function boot(): void
{
Event::listen(queueable(function (OrderPlaced $event) {
// ...
}));
}
You may also use Queue-related functions to customize the execution.
Add Trigger to respective place:-
When a user orders in your application, we need to trigger the event. Suppose the user placed an order in the application. We need to send notifications, SMS, and emails to users. You can check the following code.
use App\Events\OrderPlaced;
public function orderPlaced()
{
....
event(new OrderPlaced());
return response()->json(['status'=>true,'message'=>'Order is Placed']);
}
Update the Event Class:-
You need to update the Event class according to your requirements. An event contains essentially held information related to the event.
<?php
namespace App\Events;
use App\Models\Order;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class OrderPlaced
{
use Dispatchable, InteractsWithSockets, SerializesModels;
/**
* Create a new event instance.
*/
public function __construct(
public Order $order,
) {}
}
Define the Listener class:-
Event listeners get an instance of the event in the handle method. We can write all the business logic inside the handle method. Suppose the user has placed an order in the portal. We can send a purchase email with an invoice.
<?php
namespace App\Listeners;
use App\Events\OrderShipped;
class OrderPlacedNotification
{
/**
* Create the event listener.
*/
public function __construct()
{
// ...
}
/**
* Handle the event.
*/
public function handle(OrderShipped $event): void
{
Mail::to($event->user->email)->send(new OrderPlaced());
}
}
You may use ShouldQueue for your listeners. The dispatcher will automatically queue it.
Event Subscribers:-
Event Subscribers are classes that may subscribe to multiple events from events within the subscriber class. The subscribe method contains an event dispatcher instance.
<?php
namespace App\Listeners;
use Illuminate\Auth\Events\Login;
use Illuminate\Auth\Events\Logout;
use Illuminate\Events\Dispatcher;
class UserEventSubscriber
{
/**
* Handle user login events.
*/
public function handleUserLogin(string $event): void {}
/**
* Handle user logout events.
*/
public function handleUserLogout(string $event): void {}
/**
* Register the listeners for the subscriber.
*/
public function subscribe(Dispatcher $events): void
{
$events->listen(
Login::class,
[UserEventSubscriber::class, 'handleUserLogin']
);
$events->listen(
Logout::class,
[UserEventSubscriber::class, 'handleUserLogout']
);
}
}
Registering Event Subscribers:-
You may register subscribers using the $subscribe property on the EventServiceProvider. Check the updated EventServiceProvider file:-
<?php
namespace App\Providers;
use App\Listeners\UserEventSubscriber;
use Illuminate\Foundation\Support\Providers\EventServiceProvider as ServiceProvider;
class EventServiceProvider extends ServiceProvider
{
/**
* The event listener mappings for the application.
*
* @var array
*/
protected $listen = [
// ...
];
/**
* The subscriber classes to register.
*
* @var array
*/
protected $subscribe = [
UserEventSubscriber::class,
];
}
This post provides six steps to implement events in Laravel. Events follow an observer pattern, allowing for subscription and listening within the application. To implement events, generate events and listeners, add triggers, update event classes, register event subscribers, and update the EventServiceProvider file. Use Queue-related functions to customize execution. Register subscribers using the $subscribe property on the EventServiceProvider.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers