Your cart is currently empty!
Unlocking the Secrets: A Beginner’s Guide to Laravel Role Magic with spatie’s Enchantment
- Introduction:
- Breaking Down Role Management and Permissions:
- Step 1: Installation - Getting Started:
- Step 2: Configuration - Setting the Scene:
- Step 3: Database Setup - Laying the Groundwork:
- Step 4: Traits - Empowering Your Models:
- Step 5: Define Roles and Permissions - Simple Commands:
- Step 6: Assigning Roles and Permissions - Handing Out Titles:
- Step 7: Check Permissions - Guarding the Gates:
- Conclusion:
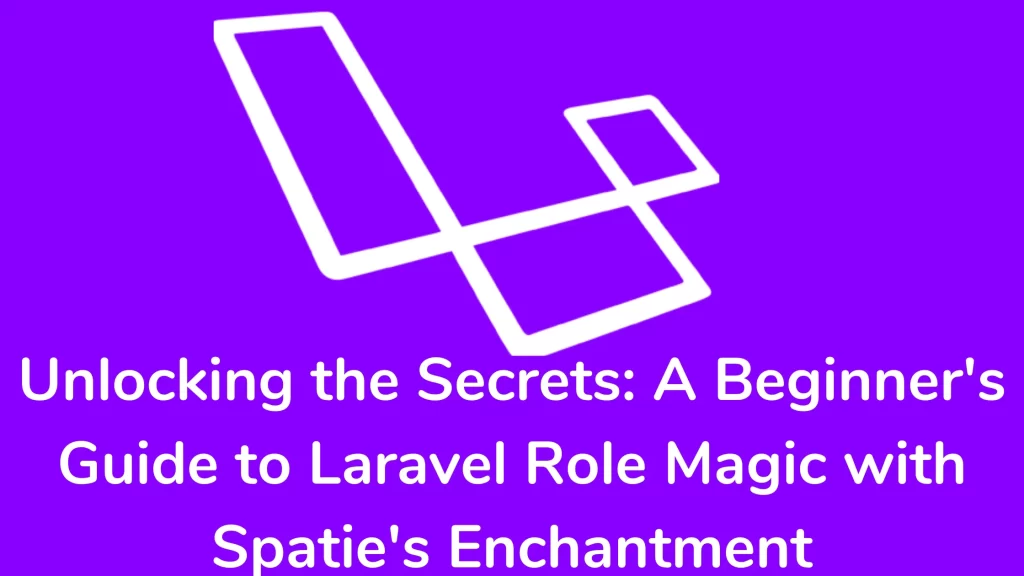
Introduction:
Understanding roles and permissions in web development is key, and Laravel simplifies this with the Spatie Laravel-Permission package. In this guide, we’ll break down the complex world of laravel role management into simple steps using Spatie’s tools.
Breaking Down Role Management and Permissions:
Imagine role management, organizing users into groups based on responsibilities and permissions as rules guiding what each group can do. Laravel’s ORM system makes managing these relationships a breeze.
Step 1: Installation – Getting Started:
Begin by installing the Spatie Laravel-Permission package with the straightforward command:
composer require spatie/laravel-permission
This sets the stage for the features you’re about to explore.
Step 2: Configuration – Setting the Scene:
Configure the package by adding a service provider and alias in config/app.php
. This step ensures smooth integration with Laravel.
'providers' => [
// Other providers...
Spatie\Permission\PermissionServiceProvider::class,
],
'aliases' => [
// Other aliases...
'Permission' => Spatie\Permission\Models\Permission::class,
],
After making these changes, run the following command to publish the package configuration:
php artisan vendor:publish --provider="Spatie\Permission\PermissionServiceProvider" --tag="config"
Step 3: Database Setup – Laying the Groundwork:
Run php artisan migrate
to create tables for roles, permissions, and their relationships, forming the backbone of your access control system.
php artisan migrate
Step 4: Traits – Empowering Your Models:
Enhance your User model by adding the HasRoles
and HasPermissions
traits from Spatie. This gives your users the tools they need.
use Spatie\Permission\Traits\HasRoles;
use Spatie\Permission\Traits\HasPermissions;
class User extends Authenticatable
{
use HasRoles, HasPermissions;
// Other model code...
}
Step 5: Define Roles and Permissions – Simple Commands:
Use Artisan commands php artisan make:role admin to define roles and php artisan make:permission create-post permissions. It’s like giving titles and powers to your users.
php artisan make:role admin
php artisan make:permission create-post
Step 6: Assigning Roles and Permissions – Handing Out Titles:
Assign roles and permissions to users with commands like:
$user = User::find(1);
$user->assignRole('admin');
$user->givePermissionTo('create-post');
This grants specific titles and powers to users.
Step 7: Check Permissions – Guarding the Gates:
Ensure the right users have the right permissions with:
if ($user->hasPermissionTo('create-post')) {
// Allow user to create a post
} else {
// Display an error message
}
Guarding access ensures a smooth user experience.
Conclusion:
Spatie Laravel-Permission is your guide in this journey. It simplifies access control in Laravel, making your application secure and user-friendly. Embrace the simplicity, and let Laravel and Spatie take your web development skills to new heights.