Your cart is currently empty!
Learn about The Laravel Controller And its Basic Concept
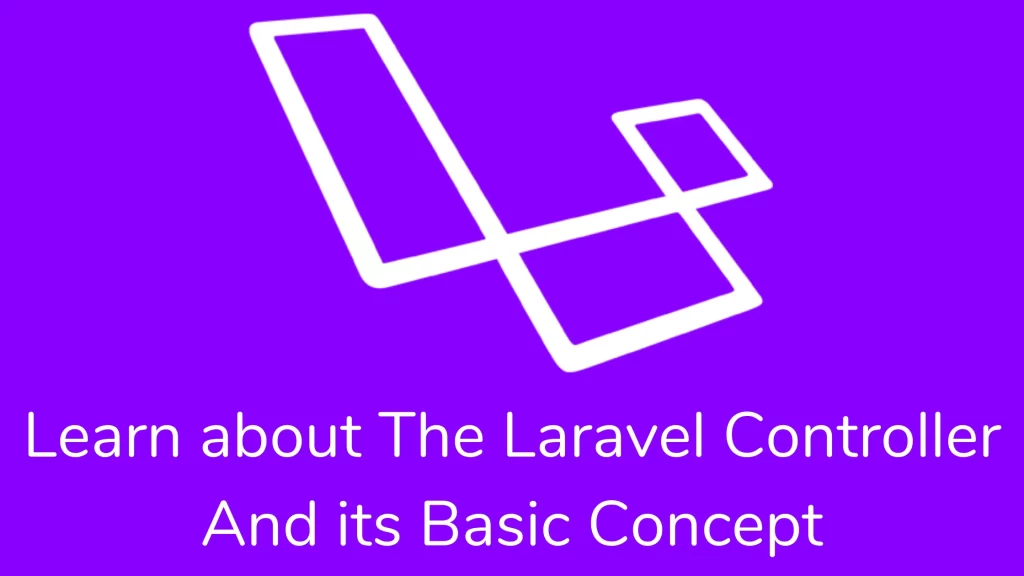
Hi guys,
You can use a controller for request-handling logic instead of defining it in the route files. Today we learn about The Laravel Controller and Its Basic Concept. It stores the controllers in the app/Http/Controllers directory.
Laravel Controllers:-
Defining Controllers:
The Controllers extend the base controller class by default in Laravel. The base controller provides a few methods. It is not mandatory to extend a base controller, but you can not access its methods. For example,
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use App\User;
class UserController extends Controller
{
/**
* Show the profile for the given user.
*
* @param int $id
* @return View
*/
public function show($id)
{
return view('user.profile', ['user' => User::findOrFail($id)]);
}
}
We can define URI inside the route file with this controller action, for example.
Route::get('user/{id}','UserController@show');
When the user requests the above route URI, the show method executes from the UserController class. The route parameter is passed to the action.
They do not need it to add the full path of the controller in the route file. When RouteServiceProvider loads the route file within a route group that contains a namespace. We need to add a class name with a function name that wants to execute.
If you have nested a controller into the App\Http\Controller directory, then you just specified the relative path of a controller. Your controller path looks like App\Http\Controllers\Admin\AdminController, write the URI given below.
Route::get('admin/dashboard','Admin\AdminController@dashboard');
You want to define a controller that contains a single method. You may name this method as ‘__invoke’ on a controller. We also know it as a single-action controller.
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use App\User;
class ShowProfile extends Controller
{
/**
* Show the profile for the given user.
*
* @param int $id
* @return View
*/
public function __invoke($id)
{
return view('user.profile', ['user' => User::findOrFail($id)]);
}
}
Inside the route file, you need to add the controller name without the function name like below:
Route::get('user/{id}', 'ShowProfile');
If you want to generate an invokable controller, then you need to pass the –invokable option to the make:controller command.
php artisan make:controller ShowProfile --invokable
Controller Middleware:-
Controller middleware has two approaches, which are below.
One approach is to assign middleware inside route files.
Route::get('profile','UserController@userProfile')->middleware('auth');
In the second approach, it is easy to define middleware inside the controllers’ constructor, and you can restrict the middleware to particular methods on the controller.
class UserController extends Controller
{
/**
* Instantiate a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('auth');
$this->middleware('log')->only('index');
$this->middleware('subscribed')->except('store');
}
}
Laravel allows you to define middleware inside the controller without defining the entire middleware class.
$this->middleware(function ($request, $next) {
// …
return $next($request);
});
Route caching:-
The route cache will drastically decrease the amount of time it takes to register all of your application’s routes. Your route registration may even be up to 100x faster. You execute the command.
php artisan route:cache
If you added a new route, you need to generate a fresh route cache. It will load the Cached Route on every request.
You want to clear the cached URI to run the following command:
php artisan route:clear
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,