Your cart is currently empty!
Mastering Efficiency: Unleashing the Power of CRUD Operations in Laravel
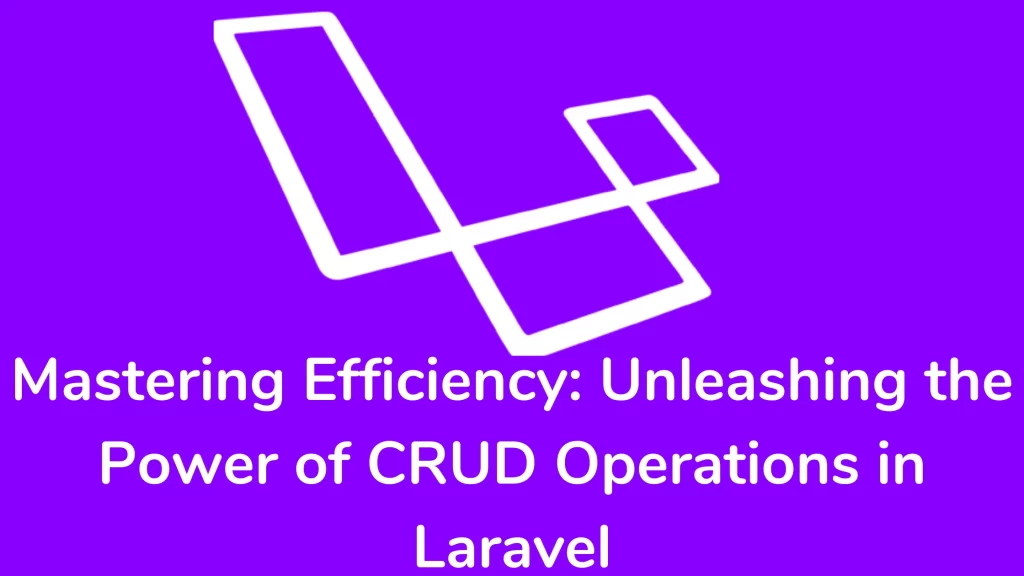
Hi Guys,
To manage structured data efficiently by providing the functionality to Create, Read, Update, and Delete records. In this article, We are studying the crud operations in laravel 9 with examples and validating user inputs in laravel.
Create a project on Laravel
Run the below command to create a laravel application.
composer create-project --prefer-dist laravel/laravel:^9.0 laravel-crud
Update the env file
Update the database credentials in the .env file.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database-name
DB_USERNAME=user-name
DB_PASSWORD=password
Make a Model and Migration
We are storing information on the database. For that, we make a migration and model using the below command.
php artisan make:model Contact -m
Now, you can check /database/migrations directory. Update the current generated file like below.
public function up()
{
Schema::create('contacts', function(Blueprint $table){
$table->id();
$table->string('name');
$table->string('email');
$table->string('mobile');
$table->timestamps();
})
}
Now, you can check the generated model and update the fillable attribute like the one below.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Elqouent\Model;
class Contact extends Model
{
use HasFactory;
protected $fillable = ['name', 'email', 'mobile'];
}
Run the migration command to generate the table.
php artisan migrate
Make a Controller
Run the below command to make the controller.
php artisan make:controller ContactController
We can see the ContactController controller and update accordingly.
<?php
namespace App\Http\Controllers;
use App\Models\Contact;
use Illuminate\Http\Request;
class ContactController extends Controller
{
public function index()
{
$contacts = Contact::paginate(10);
return view('contacts.index', compact('contacts'));
}
public function create()
{
return view('contacts.create');
}
public function store(Request $request)
{
$request->validate([
'name' => 'required',
'email' => 'required',
'mobile' => 'required'
]);
Contact::create($request->all());
return redirect()->route('contacts.index')->with('success','Contact has been created successfully');
}
public function edit($id)
{
$contact = Contact::find($id);
return view('contacts.create', compact('contact'))
}
public function update($id, Request $request)
{
$request->validate([
'name' => 'required',
'email' => 'required',
'mobile' => 'required'
]);
$contact = Contact::find($id);
$contact->update($request->all());
return redirect()->route('contacts.index')->with('message', 'Contact has been updated successfully');
}
public function destroy($id)
{
$contact = Contact::find($id);
$contact->delete();
return redirect()->route('contacts.index')->with('message', 'Contact has been deleted successfully');
}
}
Register a routes
Now, we can register routes in the web.php file.
use App\Http\Controllers\ContactController;
Route::get('/contacts', [ContactController::class, 'index']);
Route::get('/contact/create',[ContactController::class, 'create']);
Route::post('/contact/store',[ContactController::class,'store']);
Route::get('/contact/{id}/edit',[ContactController::class, 'edit']);
Route::post('/contact/{id}/update',[ContactController::class, 'update']);
Route::get('/contact/{id}/delete',[ContactController::class,'destroy']);
Create a blade views file
Make a directory inside the resources/views directory. Create directory name contacts inside the resources/views directory. Now, create two files create.blade.php and index.blade.php
Check the below code for the index file.
<html>
<head>
<title>Laravel CURD Tutorials</title>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-lg-12">
<div class="pull-left">
<h2>Laravel CRUD Operations</h2>
</div>
<div class="pull-right">
<a class="btn btn-info" href="{{ url('/contact/create')}}">Create Contact</a>
</div>
</div>
</div>
@if($message = Session::get('success'))
<div class="alert alert-success">
<p>{{$message}}</p>
</div>
@endif
<table class="table">
<tr>
<th>Sr. No.</th>
<th>Name</th>
<th>Email</th>
<th>Mobile</th>
<th>Action</th>
</tr>
@foreach($contacts as $key => $contact)
<tr>
<td>{{ $key++ }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->email }}</td>
<td>{{$contact->mobile }}</td>
<td><a href="{{url('/contact/'.$contact->id.'/edit')}}">Edit</a> <a href="{{url('/contact/'.$contact->id.'/delete')}}">Delete</a></td>
</tr>
@endforeach
</table>
{!! $contacts->links() !!}
</div>
</body>
</html>
Check the below code to create a file.
<html>
<head>
<title>Laravel CRUD Operations</title>
</head>
<body>
<div class="container">
<div class="row">
<div class="pull-left">
<h2>{{empty($contact) ? 'Add Contact': 'Update Contact'}}</h2>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{url('/contacts')}}">Back</a>
</div>
</div>
@foreach ($errors->all() as $error)
<p> {{ $error }}</p><br/>
@endforeach
@if(empty($contact))
<form action="{{url('contact/store')}}" method="POST">
@else
<form action="{{url('contact/'.$contact->id.'/update')}}" method="POST">
@endif
<div class="form-group">
<label>Contact Name</label>
<input type="text" name="name" class="form-control" value="{{ empty($contact->name) ? '' : $contact->name }}">
<div class="form-group">
<label>Contact Email</label>
<input type="email" name="email" class="form-control" value="{{ empty($contact->email) ? '' : $contact->email }}">
<div class="form-group">
<label>Contact Mobile</label>
<input type="text" name="mobile" class="form-control" value="{{ empty($contact->mobile) ? '' : $contact->mobile }}">
<button type="submit" class="btn btn-primary">{{ empty($contact) ? 'Save': 'Update' }}</button>
</div>
</form>
</div>
</body>
</html>
Run the Development Server
To start the laravel application, you need to run the below command.
php artisan serve
Then open your browser and hit the following URL on it.
http://127.0.0.1:8000/contacts
The main point of the given text is to provide a step-by-step guide on how to create a CRUD (Create, Read, Update, Delete) operation app in Laravel 9, including creating a project, updating the environment file, making a model and migration, creating a controller, registering routes, creating blade views files, and running the development server.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers