Your cart is currently empty!
Laravel Middleware and its Interesting Facts
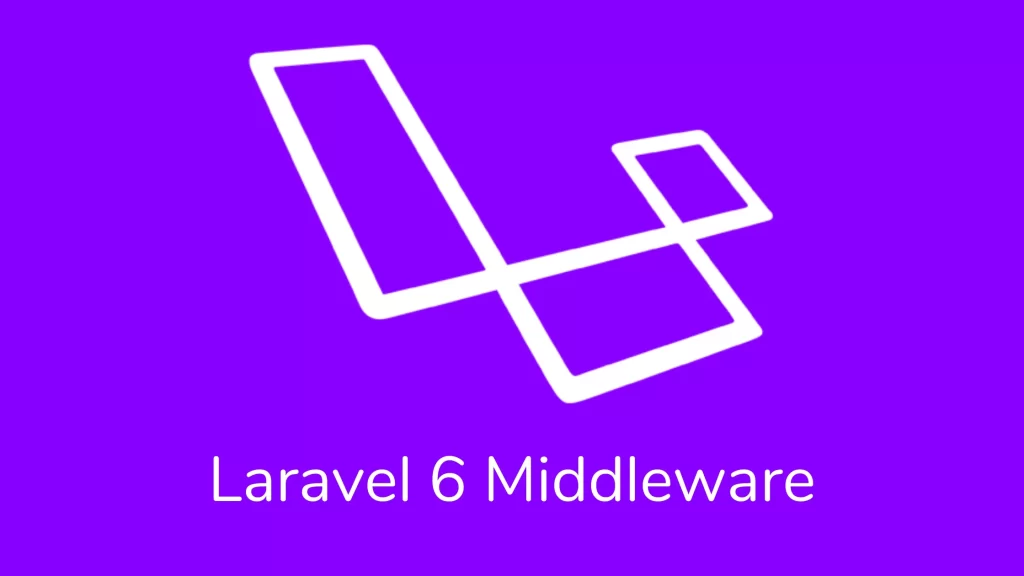
Hi guys,
Today we are studying the laravel middleware. So Don’t skip this article you get the best knowledge. Stay connected!
Middleware is the best way to filter HTTP requests for your application. Laravel contains some middleware by default. For example, The Middleware verifies the user of the application has authenticated. If the user has not validated, The Middleware will redirect to the login page. If the user has validated, it will allow proceeding further. All middleware was discovered in the App/Http/Middleware directory.
You can create your middleware using the artisan command:
php artisan make:middleware AdminMiddleware
AdminMiddleware file has spawned in the App/Http/Middleware directory. Only admin role users can access the route. Otherwise, it will redirect to the login page.
<?php
namespace App\Http\Middleware;
use Closure;
use Auth;
class AdminMiddleware
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
if(auth::check() && Auth::user()->role == 1){
return $next($request);
}
else {
return redirect()->route('login');
}
}
}
As you can see, we are checking the role of the user. If the above condition is true, then the middleware will return an HTTP redirect to the client. Otherwise, it will redirect to the login page.
Laravel Middleware
Laravel Middleware runs before or after a request depending on the middleware itself. The Middleware performs some action on a request before handover to the application. Below, middleware acts as a before Middleware.
<?php
namespace App\Http\Middleware;
use Closure;
class BeforeMiddleware
{
public function handle($request, Closure $next)
{
// Perform action
return $next($request);
}
}
First, the request is handed over to the application and then passed to the middleware. For example,
<?php
namespace App\Http\Middleware;
use Closure;
class AfterMiddleware
{
public function handle($request, Closure $next)
{
$response = $next($request);
// Perform action
return $response;
}
}
Registering Middleware:-
Global Middleware:
If you want to run middleware on every request of your application. Write the middleware class in the $middleware property of your app/Http/Kernel.php file.
Assigning Middleware to a route:
If you would like to assign middleware to a specific URL, write the class into $route middleware property with a key in your app/Http/Kernel.php file. The code from the kernel file.
protected $routeMiddleware = [
'auth' => \App\Http\Middleware\Authenticate::class,
'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class,
'bindings' => \Illuminate\Routing\Middleware\SubstituteBindings::class,
'cache.headers' => \Illuminate\Http\Middleware\SetCacheHeaders::class,
'can' => \Illuminate\Auth\Middleware\Authorize::class,
'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class,
'password.confirm' => \Illuminate\Auth\Middleware\RequirePassword::class,
'signed' => \Illuminate\Routing\Middleware\ValidateSignature::class,
'throttle' => \Illuminate\Routing\Middleware\ThrottleRequests::class,
'verified' => \Illuminate\Auth\Middleware\EnsureEmailIsVerified::class,
'admin' => \App\Http\Middleware\AdminMiddleware::class
];
After defining middleware in the kernel, you can use the middleware method to filter the routes. Some of the example,
Route::get('admin/profile', function () {
//
})->middleware('auth');
Assign multiple middleware to a route. For Example,
Route::get('/', function () {
//
})->middleware('first', 'second');
Middleware group:
You want to create a group of middleware under a single key to make it easier to assign to routes. You may do this using the $middlewareGroups property of a kernel. Laravel already contains a web and API middleware group. The below code is from the HTTP kernel file.
protected $middlewareGroups = [
'web' => [
\App\Http\Middleware\EncryptCookies::class,
\Illuminate\Cookie\Middleware\AddQueuedCookiesToResponse::class,
\Illuminate\Session\Middleware\StartSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\VerifyCsrfToken::class,
\Illuminate\Routing\Middleware\SubstituteBindings::class,
],
'api' => [
'throttle:60,1',
'auth:api',
],
];
The Middleware group makes it easy to assign middleware to a route at once. For example,
Route::group(['middleware' => ['web']], function () {
//
});
Route::middleware(['web', 'subscribed'])->group(function () {
//
});
Sorting Middleware:
You want to execute middleware in a specific order when they have been assigned to routes. You can set an order for the middleware in the $middlewarePripority property in an HTTP kernel file. The below code is from the kernel.
protected $middlewarePriority = [
\Illuminate\Session\Middleware\StartSession::class,
\Illuminate\View\Middleware\ShareErrorsFromSession::class,
\App\Http\Middleware\Authenticate::class,
\Illuminate\Session\Middleware\AuthenticateSession::class,
\Illuminate\Routing\Middleware\SubstituteBindings::class,
\Illuminate\Auth\Middleware\Authorize::class,
];
Middleware Parameters:-
You can receive additional parameters. For example, The Middleware checks the role of an authenticated user before the process.
<?php
namespace App\Http\Middleware;
use Closure;
class CheckRole
{
/**
* Handle the incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @param string $role
* @return mixed
*/
public function handle($request, Closure $next, $role)
{
if (! $request->user()->hasRole($role)) {
// Redirect…
}
return $next($request);
}
}
We can define the middleware name and parameters with ‘:’. If you want to pass multiple parameters to the middleware, then you can pass comma-separated parameters.
Route::put('post/{id}', function ($id) {
//
})->middleware('role:editor');
Terminable Middleware:-
Some task has to be completed after an HTTP response has been sent to the browser. If you define a terminate method on your middleware and your web server is using FastCGI, the terminate method automatically runs.
<?php
namespace Illuminate\Session\Middleware;
use Closure;
class StartSession
{
public function handle($request, Closure $next)
{
return $next($request);
}
public function terminate($request, $response)
{
// Store the session data
}
}
The terminate method has two parameters: request and response. When calling the terminate method on your middleware, Laravel will resolve a fresh instance of the middleware from the service container.
If you want to use the same instance for the handle and the terminate method, then you register the middleware with the container using the container’s singleton method.
use App\Http\Middleware\TerminableMiddleware;
/**
* Register any application services.
*
* @return void
*/
public function register()
{
$this->app->singleton(TerminableMiddleware::class);
}
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,