Your cart is currently empty!
How to make a controller and its route in Laravel?
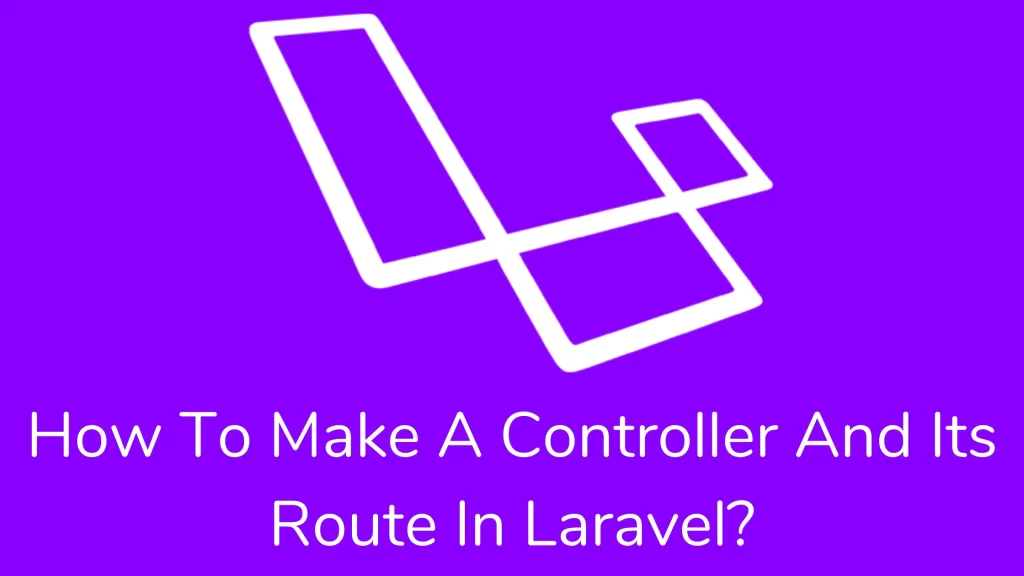
Hi guys,
Today we are studying How to make a controller and its routes in Laravel. In previous articles, we have studied the basics of Controller in Laravel. We can easily create a controller given below:
php artisan make:controller CarController --resource
The above command creates a basic structure of a controller with all resource operations. Then you can use a resource method to create all resource routes.
Route::resource('car','CarController');
Also, you can pass an array of multiple resource controllers to resource method like below:
Route::resource([
'car'=>'CarController',
'post'=>'PostController'
]);
Actions Handled By Resource Controller
Verb | URI | Action | Route Name |
---|---|---|---|
GET | /p osts | index | posts.index |
GET | /posts/create | create | posts.create |
POST | /posts | store | posts.store |
GET | /posts/{post} | show | posts.show |
GET | /posts/{post}/edit | edit | posts.edit |
PUT/PATCH | /posts/{post} | update | posts.update |
DELETE | /posts/{post} | destroy | posts.destroy |
If you are using route model binding it will be a type-hint inside a controller method. You can use the — model option when generating the controller.
php artisan make:controller CarController --resource --model=Car
HTML forms do not make PUT, PATCH, and DELETE requests. You need to create the _method field in the HTML form or you can use the @method function inside the form like below.
<form action="/foo/bar" method="POST">
@method('PUT')
</form>
Partial Resource Routes:
You can also manage operations of the resource controller using only, except functions.
Route::resource('photos', 'PhotoController')->only([
'index', 'show'
]);
Route::resource('photos', 'PhotoController')->except([
'create', 'store', 'update', 'destroy'
]);
API Resource Routes
The apiResource method excludes create and edit operations because these operations return the view page. It will set up all remaining routes.
Route::apiResourc (('photos', 'PhotoController');
You can pass an array of multiple resource controller to apiResource method like below:
Route::apiResources([
'photos' => 'PhotoController',
'posts' => 'PostController'
]);
You can quickly generate an API resource controller that excludes the create and edit method because the create and edit operations return a view. Add –api option to make:controller command.
php artisan make:controller API/PhotoController --api
Nested Resources:-
A Post may have multiple comments. That time nested resources come into the picture.
Route::resource('posts.comments','PostCommentController');
This route will register a nested resource that accessed with URI like below :
/posts/{post}/comments/{comment}
Shallow Nesting:
It is unnecessary to have a post id and comment id within a URI but a comment id is already a unique identifier. (If comment id is auto-increment key).
Route::resource('posts.comments', 'CommentController')->shallow();
Verb | URI | Action | Route Name |
---|---|---|---|
GET | /posts/{post}/comments | index | posts.comments.index |
GET | /posts/{post}/comments/create | create | posts.comments.create |
POST | /posts/{post}/comments | store | posts.comments.store |
GET | /comments/{comment} | show | comments.show |
GET | /comments/{comment}/edit | edit | comments.edit |
PUT/PATCH | /comments/{comment} | update | comments.update |
DELETE | /comments/{comment} | destroy | comments.destroy |
Naming Resource Route:-
By default, Resource controller actions have a route name. You can override these names by passing the name array.
Route::resource('photos', 'PhotoController')->names ([
'create' => 'photos.build'
]);
Naming Resource Route Parameters:-
You can easily override this on a per resource basis by using the parameters method. The array passed into the parameters method should be an associative array of resource names and parameter names.
Route::resource('users', 'AdminUserController')->parameters([
'users' => 'admin_user'
]);
Above resource route will generate given below:
/users/{admin_user}
Localizing Resource URIs:-
By default, Laravel makes resource URI using English verbs. You want to localize URIs. You can use the resource Verbs() method. We should make these changes inside the AppServiceProvider boot method.
<?php
use Illuminate\Support\Facades\Route;
/**
Bootstrap any application services.
*
@return void
*/
public function boot()
{
Route::resourceVerbs([
'create' => 'crear',
'edit' => 'editar',
]);
}
You can define a localized resource route like below :
Route::resource('fotos', 'PhotoController');
Supplementing Resource Controllers-
If you want to add an extra route to a controller, that does not exist in a default route. You must write extra route before Route::resource method like below:
Route::get('/user/photo','UserController@photo');
Route::resource('user','UserController');
Dependency Injection & Controllers:-
Constructor Injection:
You can type-hint any dependencies your controller may need in its constructor. It will resolve this dependency when the controller instance is injected.
<?php
namespace App\Http\Controllers;
use App\Repositories\UserRepository;
class UserController extends Controller
{
/**
* The user repository instance.
*/
protected $users;
/**
* Create a new controller instance.
*
* @param UserRepository $users
* @return void
*/
public function __construct(UserRepository $users)
{
$this->users = $users;
}
}
Injecting your dependencies into your controller may provide better testability.
Method Injection:
You can also inject dependencies on your controller’s method. So the most common example that is injecting a Request dependency on the controller’s method.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
/**
* Store a new user.
*
* @param Request $request
* @return Response
*/
public function store(Request $request)
{
$name = $request->name;
//
}
}
If your controller needs extra input from the route parameter. you can write a list of a parameter with other dependencies
Route::put('user/{id}', 'UserController@update');
You may still type-hint the Illuminate\Http\Request
and access your id
parameter by defining your controller method:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
/**
* Update the given user.
*
* @param Request $request
* @param string $id
* @return Response
*/
public function update(Request $request, $id)
{
//
}
}
I am pretty sure this article (How to make a controller and its route in Laravel?) it help you a better understanding of the controller and its routes. I have followed this link for this article. If you have any doubts, then comment below then I will reply as per as possible.
Thank you for reading this article. Please share this article with your friend circle who wants to enhance their skill in the Laravel framework. That’s it for the day. Stay Connected!
Cheers,