Your cart is currently empty!
Some interesting things about Request in laravel
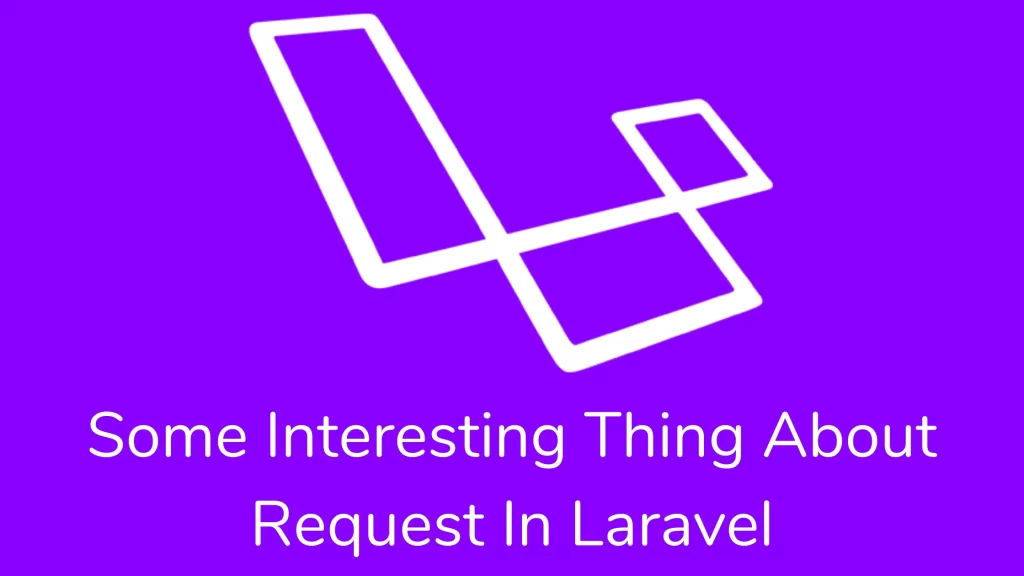
Hi guys,
Are you interested in learning about Request in Laravel and are there some interesting things that you can use on your project? You will have greater knowledge of requests in Laravel.
Accessing the Request in Laravel:-
You can access the current HTTP request using dependency injection. You should type Request as an argument for the controller’s method. The service container will automatically inject the incoming HTTP request.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class UserController extends Controller
{
/**
* Store a new user.
*
* @param Request $request
* @return Response
*/
public function store(Request $request)
{
$name = $request->input('name');
}
}
Dependency injection & Route parameters:
You want an access parameter which dynamically generated. You can pass the parameters list as arguments to it. For example,
Route::get('user/{id}','UserController@get');
The controller’s method looks like
public function get( $id)
{
//
}
Request Path & Method:-
The Request contains many methods for examining the HTTP request.
Retrieving the request path:
The path() method returns the requested path information.
$uri = $request->path();
Suppose you are visiting the URL (http://yourdomain.com/bar/foo), and the path method will return bar/foo.
The is() method is confirming request path matches a pattern, and you can * character as a wildcard.
if ($request->is('admin/*')) {
//
}
The url() method shows the URL without the query string. If your visiting URL https://yourdomain.com/foo/bar?name=james
$url = $request->url();
// output - https://yourdomain.com/foo/bar
The fullUrl() method shows a URL with a query string.
$url = $request->fullUrl();
// output - https://yourdomain.com/foo/bar?name=james
Retrieving the Request method:
The method will return the HTTP verb for the HTTP Request. The isMethod() method is to verify request matches the string.
$method = $request->method();
if ($request->isMethod('post')) {
//
}
Retrieving Input:-
you will get all the input data as an array using all methods:
$input = $request->all();
Retrieving an input value:
You may access specific input data using the Request instance. The input method may retrieve user input.
$name = $request->input('name');
you can pass a default value as the second argument to an input method
$name = $request->input('name','sally');
when working with forms that contain array inputs, use ‘dot’ notation to access the arrays
$name = $request->input('products.*.name');
You can use the input method with no argument. You will get all input values in an associative array.
$input = $request->input();
Retrieving input from query string:
The input method returns all input values with query parameters. The query method will only retrieve values from the query string.
$name = $request->query('name');
If the requested query string value not exist, you can pass the second argument to the query method. It looks like the default value.
$name = $request->query('name', 'Helen');
You can use the query method with no argument. You will get all query string values in an associative array.
$query = $request->query();
Dynamic Properties:
You can access the forms field directly with no method using the Request instance.
$name = $request->name;
Laravel looks at it first on request payload. If it does not exist, then it searches inside route parameters.
Access JSON data:
You may access JSON data via the input method if you set the request header Content-Type: ‘application/json’. You use the ‘dot’ operator to access the value.
$name = $request->input('user.name');
Retrieving Boolean Input Values:
As an input method, you will truth values from checkboxes. But we can better way to deal with truth values using the boolean method. It returns true when the certainty value comes. Otherwise, it will return false.
$archived = $request->boolean('archived');
Retrieving A Portion Of Input Data:
If you want to get some input fields from the form, you may use only and except methods. You can pass a single array or list of parameters.
$input = $request->only(['username','password']);
$input = $request->except('credit_card');
Determining If the input value is present:
You can use the has method to determine whether a value is present or not. If the value exists, then it returns true, otherwise returns false. Also, you can pass the single array as an argument.
if($request->has('name')) {
}
The hasAny method returns true if any specified values are present
if($request->hasAny(['name','email'])) {
}
If a value is present on the HTTP request and is not empty, you use a filled method. If a key does not exist on an HTTP request, then you use the missing method.
if($request->filled('name')) {
}
if($request->missing('name')) {
}
Old Input:-
Laravel’s feature is repopulating forms after detecting validation errors.
Flashing Input session:
The flash method will flash the current input to the session. So it is available for the next HTTP request.
$request->flash();
The flashOnly and flashExcept methods flash a subset of the requested data to the session.
$request->flashOnly(['name','email']);
$request->flashExcept('password');
Flashing input then Redirecting:
You want to flash data to the session and redirect to the previous page. You can easily do this with the withInput method.
return redirect('form')->withInput();
Retrieving Old method:
Retrieving value from the previous HTTP request using the old method of request instance.
$name = $request->old('name');
Also, you can retrieve the old value inside the blade template using the old() helper.
Cookies:-
Retrieving cookies from requests:
All cookies are encrypted and signed by authenticated code by the Laravel framework. To retrieve a cookie value from the HTTP request using the cookie method.
$value = $request->cookie('name');
Attaching cookies to response:
You can attach cookies to the response using the cookie method. you should pass the name, value, no of minutes
return response('Hello')->cookie(
'name','value',$minutes, $path, $domain, $secure, $httpOnly
);
The queue method accepts a Cookie instance or the arguments needed to create a cookie method.
Cookie::queue(Cookie::make('name','value',$min));
Cookie::queue('name','value',$min);
You use the global cookie helper function to create cookies. These cookies are not sent to the client unless we attach them to a response instance.
$cookie = cookie('name', 'value', $min);
return response('Hello')->cookie($cookie);
Files:-
Retrieving uploaded files:
You can access uploaded files using the File method of request instance. The file method returns the object of the UploadedFile class.
$file = $request->file('photo');
$file = $request->photo;
You want to check file is present on the HTTP request using the hasFile method.
if($request->hasFile('photo')) {
}
If you want to check whether the file has no problem while uploading a file via the isValid method.
if($request->hasFile('photo')->isValid()) {
}
There are many methods for accessing file properties. The extension() method will get file extension.
$path = $request->photo->path();
$extension = $request->photo->extension();
Storing Uploaded Files:-
The UploadedFile class has a store method that will move an uploaded file to one disk. The store method also accepts an optional second argument for the disk name that should store the file.
$path = $request->photo->store('images');
$path = $request->photo->store('images', 's3');
If you don’t want a file name to be automatically generated, you can use the storeAs method which accepts the path, filename, and disk name as its arguments:
$path = $request->photo->storeAs('images', 'filename.jpg');
$path = $request->photo->storeAs('images', 'filename.jpg', 's3');
Configuring Trusted Proxies:-
When running your applications behind a load balancer that terminates TLS / SSL certificates, you may notice your application sometimes does not generate HTTPS links. You can add proxies to your TrustProxies middleware inside the $proxies property.
<?php
namespace App\Http\Middleware;
use Fideloper\Proxy\TrustProxies as Middleware;
use Illuminate\Http\Request;
class TrustProxies extends Middleware
{
/**
* The trusted proxies for this application.
*
* @var string|array
*/
protected $proxies = [
'192.168.1.1',
'192.168.1.2',
];
/**
* The headers that should be used to detect proxies.
*
* @var string
*/
protected $headers = Request::HEADER_X_FORWARDED_ALL;
}
If you do not know the IP address of the load balancing server you can assign * to proxies property.
protected $proxies = '*';
I am pretty sure this article (Some Interesting Things About Requests In Laravel) it help you a better understanding of the requests in Laravel. I have followed this link for this article. If you have any doubts, then comment below then I will reply as per as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,