Your cart is currently empty!
Important Points while making View in Laravel
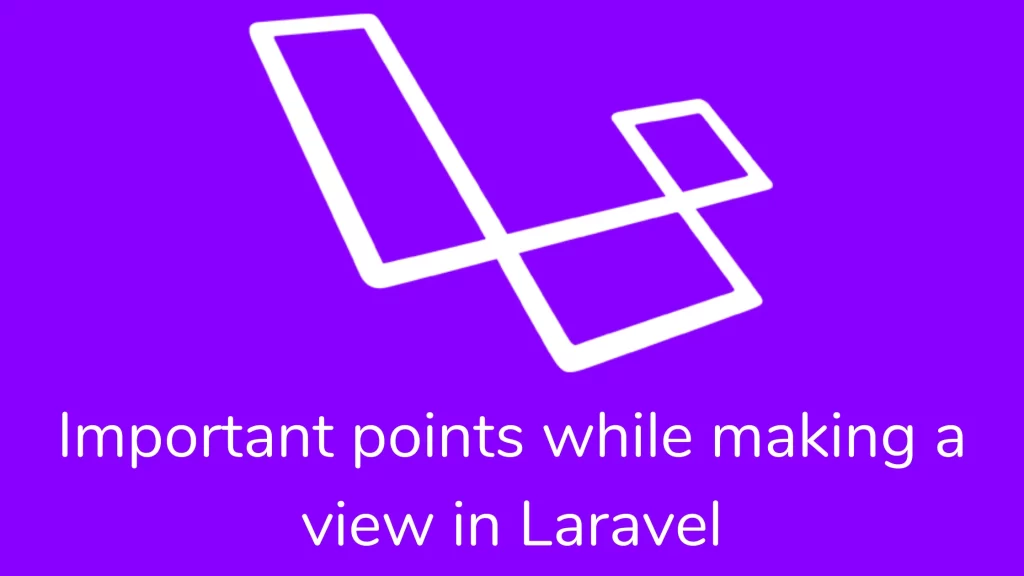
Hi guys,
Today we are to learn how to create a view and how to pass data to a view in Laravel. Also, you can manage a view using view composers and view creators.
Creating Views:-
The view separates your controller/application logic from your presentation logic in the resource/view directory. You may call the view helper method to display blade content.
Route::get('/', function () {
return view('greeting', ['name' => 'James']);
});
The first argument passed to the view function corresponds to the name of the view file. The second argument is an array of data.”Dot” notation may reference nested views. The dashboard file is inside the admin folder and the admin folder is inside the view directory.
return view('admin.dashboard', $data);
Determining If A View Exists
You want to check whether the view exists. In that case, use the exists() method from the View facade.
use Illuminate\Support\Facades\View;
if(View::exists('user.email'))
{
}
Creating The First Available View
Using the First method, you may create the first view that appears in a given array of views. This method may be useful if your application or package allows views to be customized or overwritten:
return view()->first(['custom.email','email'],$data);
Passing Data To Views:-
You may pass on an array of data to views as given below.
return view('greetings',['name'=>'Rocky']);
You can also pass the individual data to the view using with() function, which is displayed below.
return view('greeting')->with('name','Rocky');
Sharing data with All views:
Sometimes, you want a piece of data across all the views of your application. In that case, the share method comes into the picture. You need to add the share method inside the boot method in AppServiceProvider or create a new service provider.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\View;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
View::share('key', 'value');
}
}
View Composers:-
View composers are callback or class methods that have called when a view is displayed. Suppose, You want to bind a view all the time when that view is published. You can organize that into a single location. Laravel does not include a default directory for view composers. You are free to establish them however you wish.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\View;
use Illuminate\Support\ServiceProvider;
class ViewServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
// Using class based composers...
View::composer(
'profile', 'App\Http\View\Composers\ProfileComposer'
);
// Using Closure-based composers...
View::composer('dashboard', function ($view) {
//
});
}
}
In Class-based composers, you need to create a Composer class with the compose() method.
<?php
namespace App\Http\View\Composers;
use App\Repositories\UserRepository;
use Illuminate\View\View;
class ProfileComposer
{
/**
* The user repository implementation.
*
* @var UserRepository
*/
protected $users;
/**
* Create a new profile composer.
*
* @param UserRepository $users
* @return void
*/
public function __construct(UserRepository $users)
{
// Dependencies automatically resolved by service container...
$this->users = $users;
}
/**
* Bind data to the view.
*
* @param View $view
* @return void
*/
public function compose(View $view)
{
$view->with('count', $this->users->count());
}
}
Before it renders a view, the View instance calls the composer’s compose method. You may also add with() function to bind data to that view.
Attaching a composer to multiple views:
You may attach a view composer with multiple views in an array format.
View::composer(
['profile','dashboard'],
'App\Http\View\Composers\ViewComposer'
);
The composer() method also accepts the * character as a wildcard, allowing you to attach a composer to all views.
View::composer('*', function($view){
});
View Creators:-
View creators are very similar to view composers. Before the view is about to perform creator method is implemented immediately.
View::creator('profile','App\Http\View\Creators\ProfileCreator');
I am pretty sure this article (Important points while making view in Laravel) it help you a better understanding of the view in Laravel. I have followed this link for this article. If you have any doubts, then comment below then I will reply as per as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,