Your cart is currently empty!
How to write Log inside controllers in Laravel?
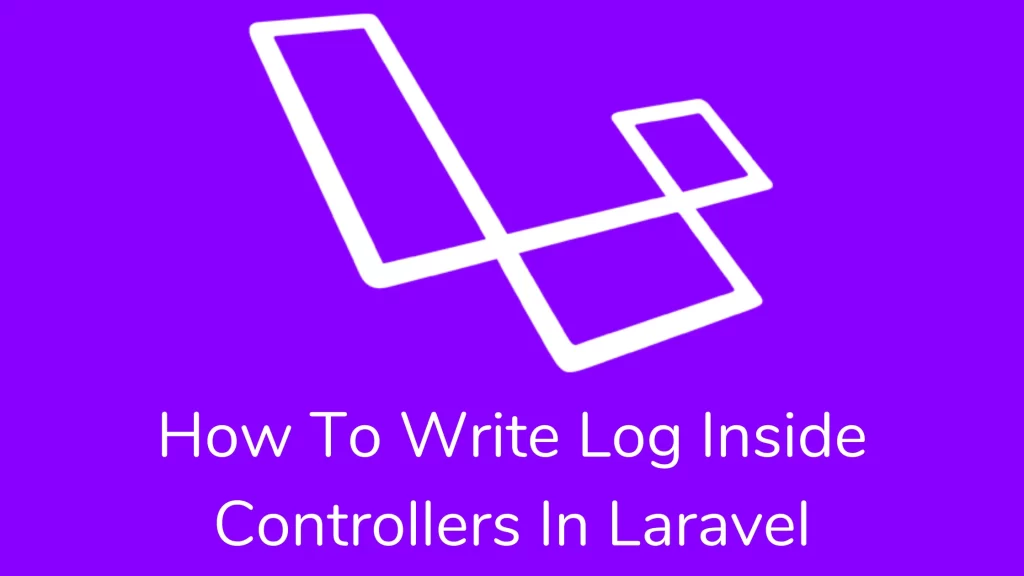
Hi guys,
Today, we’ll explore Laravel’s How To Write Log Inside Controllers in Laravel. Laravel logs messages using the RFC5424 log level, and how can you use Log Facade to create any type of log? We’ll also talk about how to create your monologue channel.
Laravel provides a robust logging service that allows you to log messages to files. Laravel uses a monolog library which is simply the logging procedure. Also, you can customize the log handling in your application.
Configuration:-
You configure the logging system to your application inside your config/logging.php file. By default, Laravel sets the channel to stack channel. A stack channel can add multiple log channels into a single channel. So it looks like below:
'stack' => [
'driver' => 'stack',
'name' => 'channel-name',
'channels' => ['single', 'slack'],
],
The above stack channel has two other channels inside the channels option, single and slack. When logging messages, both channels will log the message.
Available Channel Drivers-
Name | Description |
stack | A wrapper to facilitate creating “multi-channel” channels |
single | A single file or path based logger channel (StreamHandler ) |
daily | A RotatingFileHandler based Monolog driver which rotates daily |
slack | A SlackWebhookHandler based Monolog driver |
papertrail | A SyslogUdpHandler based Monolog driver |
syslog | A SyslogHandler based Monolog driver |
errorlog | A ErrorLogHandler based Monolog driver |
monolog | A Monolog factory driver that may use any supported Monolog handler |
custom | A driver that calls a specified factory to create a channel |
- The paper trail channel requires the URL and port configuration options.
- The slack channel requires a URL configuration option. This URL Should match the URL of an incoming webhook that you have configured for your Slack team.
The channel has the option “level” that determines the minimum level of the message that is to be logged.
Log level:-
Emergency, critical, alert, error, warning, notice, info, and debug are the RFC5424 log levels available in Monologs.
Numerical Severity
Code
0 Emergency: the system is unusable
1 Alert: Action has to take immediately
2 Critical: critical conditions
3 Error: error conditions
4 Warning: warning conditions
5 Notice: Normal but significant condition
6 Informational: informational messages
7 Debug: debug-level messages
Suppose we log the message using the debug method:
Log::debug('An informational message.');
It will write the message to the system in a single channel. If the error message is critical or above, it will ping the slack channel and write it into the system log.
Log::emergency('The system is down!');
Writing Log messages:-
It is the easiest way to write logs into your application. I have listed below how to write the error message in your application.
Log::emergency($message);
Log::alert($message);
Log::critical($message);
Log::error($message);
Log::warning($message);
Log::notice($message);
Log::info($message);
Log::debug($message);
The message has been written to the default log channel as we defined in the config/logging.php file.
How to write Log inside a controller?:-
There are two steps to write a log inside the Controller.
step 1 – Add Log Class from facades
use Illuminate\Support\Facades\Log;
step 2 – Write method from Log class
Log::info('Showing user profile for user: '.$id);
after completing the steps, it looks like below:
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use App\User;
use Illuminate\Support\Facades\Log;
class UserController extends Controller
{
/**
* Show the profile for the given user.
*
* @param int $id
* @return Response
*/
public function showProfile($id)
{
Log::info('Showing user profile for user: '.$id);
return view('user.profile', ['user' => User::findOrFail($id)]);
}
}
Contextual information:
You may pass an array to log methods. They will display it on the log message. You can write like below:
Log::info('User failed to login.', ['id' => $user->id]);
Writing to specific channels:
You might log messages to another channel then you use the channel method of the Log facade to retrieve the value from the configuration file.
Log::channel('slack')->info('Something happened!');
You may require the stack method to write a log message to multiple channels.
Log::stack(['single', 'slack'])->info('Something happened!');
How to make custom monolog channels?
Suppose you wish to complete control of the log channel. In that case, you can make a custom monolog channel by implementing the FormatterInterface interface.
We add a tap option inside the channel. The tap array contains a list of classes that implemented FormatterInterface.
'single' => [
'driver' => 'single',
'tap' => [App\Logging\CustomizeFormatter::class],
'path' => storage_path('logs/laravel.log'),
'level' => 'debug',
],
After defining the tap option inside your channel. We have to create a class that changes your monolog instance. This class only needs a single method: __invoke, which receives an Illuminate\Log\Logger instance. So it looks like below:
<?php
namespace App\Logging;
use Monolog\Formatter\LineFormatter;
class CustomizeFormatter
{
/**
* Customize the given logger instance.
*
* @param \Illuminate\Log\Logger $logger
* @return void
*/
public function __invoke($logger)
{
foreach ($logger->getHandlers() as $handler) {
$handler->setFormatter(new LineFormatter(
'[%datetime%] %channel%.%level_name%: %message% %context% %extra%'
));
}
}
}
Note:- The Service Container has invoked all taps.
Creating channels via Factories:-
You wish to complete control of monolog instantiation and configuration. In the logging.php file, you can create custom driver types. You may use the configuration option. Laravel will invoke a monolog instance.
'channels' => [
'custom' => [
'driver' => 'custom',
'via' => App\Logging\CreateCustomLogger::class,
],
],
After adding a custom channel, you can create a class that will make your monolog instance. this class should contain the __invoke a method that returns a monolog instance.
<?php
namespace App\Logging;
use Monolog\Logger;
class CreateCustomLogger
{
/**
* Create a custom Monolog instance.
*
* @param array $config
* @return \Monolog\Logger
*/
public function __invoke(array $config)
{
return new Logger(…);
}
}
Creating Monolog Handler channels:
You want to create a monolog driver with an instance of the specified handler.
The handler option is used to specify which handler will instantiate using a monolog driver. If the handler needs constructor parameters, you may define using with option.
'logentries' => [
'driver' => 'monolog',
'handler' => Monolog\Handler\SyslogUdpHandler::class,
'with' => [
'host' => 'my.logentries.internal.datahubhost.company.com',
'port' => '10000',
],
],
Monolog uses LineFormatter as the default format. You may customize the log message by passing formats and formatter_with options.
'browser' => [
'driver' => 'monolog',
'handler' => Monolog\Handler\BrowserConsoleHandler::class,
'formatter' => Monolog\Formatter\HtmlFormatter::class,
'formatter_with' => [
'dateFormat' => 'Y-m-d',
],
],
If you do not want to change the format, then you may set format to the default.
'newrelic' => [
'driver' => 'monolog',
'handler' => Monolog\Handler\NewRelicHandler::class,
'formatter' => 'default',
],
I hope this article (how to write log inside the controller in laravel) helps you to better understand the create custom monolog channels. For this article, I followed this link. If you have questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,