Your cart is currently empty!
how to deal with exceptions in Laravel
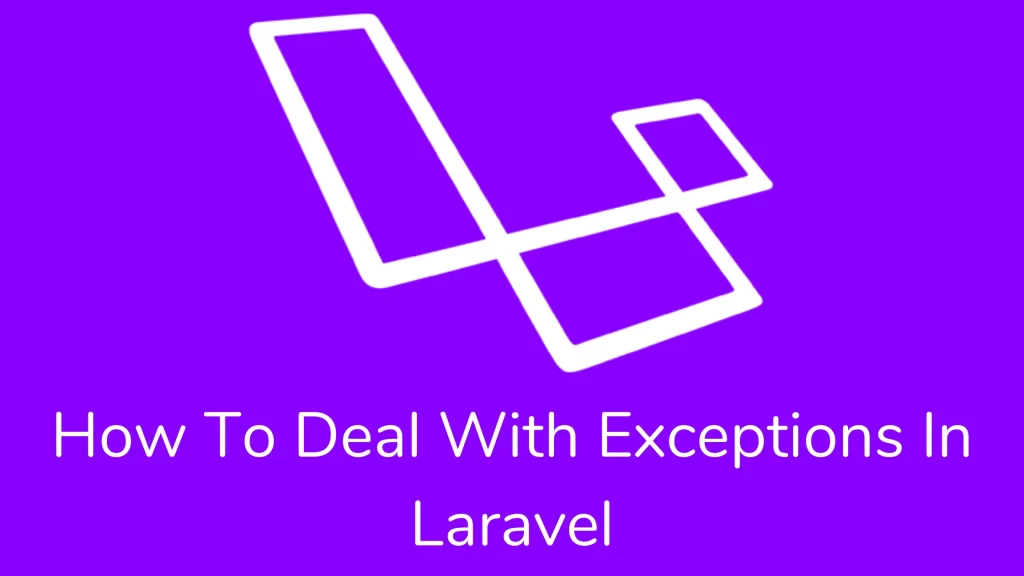
Hi guys,
We’ll look at how to deal with exceptions in Laravel today. In addition, exceptions are notified and provided. We may personalize the error page and deliver particular HTTP Status codes based on the design of your application.
Laravel has already configured error and exception handling for you. You can display error information to the user using the debug option inside the app.php file. You can set the APP_DEBUG variable inside your .env file.
Exception Handler:-
The App\Exceptions\Handler class handles all exceptions. There are two methods in this class: report and render. The report function logs the exception and sends it back to the base class. You may use a flare, or sentry, to communicate the exception to a third-party program. You wish to use the instance operator to report unique exceptions in various ways, as seen below:
/**
Report or log an exception.
*
This is a great spot to send exceptions to Flare, Sentry, Bugsnag, Etc.
*
@param \Throwable $exception
@return void
*/
public function report(Throwable $exception)
{
if ($exception instanceof CustomException) {
//
}
parent::report($exception);
}
Global Log Context:
You want to write a log that contains the current user ID along with an exception message. In that case, you need to override the context method from the App\Exceptions\Handler class.
/**
Get the default context variables for logging.
*
@return array
*/
protected function context()
{
return array_merge(parent::context(), [
'foo' => 'bar',
]);
}
The report Helper:
The report helper function allows you to report an issue without having to go to the error page.
public function isValid($value)
{
try {
// Validate the value…
} catch (Throwable $e) {
report($e);
return false;
}
}
The $dontReport variable contains an array of exception types that ignore inside the Handler class.
/**
A list of the exception types that should not report.
*
@var array
*/
protected $dontReport = [
\Illuminate\Auth\AuthenticationException::class,
\Illuminate\Auth\Access\AuthorizationException::class,
\Symfony\Component\HttpKernel\Exception\HttpException::class
];
The Render method:
The render method handles an HTTP response that is a setback to the user. You also check the exception type and return your custom view.
/**
Render an exception into an HTTP response.
*
@param \Illuminate\Http\Request $request
@param \Throwable $exception
@return \Illuminate\Http\Response
*/
public function render($request, Throwable $exception)
{
if ($exception instanceof CustomException) {
return response()->view('errors.custom', [], 500);
}
return parent::render($request, $exception);
}
Reportable & Renderable Exceptions:
You may define the report and render method inside your custom exception class. The framework will automatically call this.
<?php
namespace App\Exceptions;
use Exception;
class RenderException extends Exception
{
/**
* Report the exception.
*
* @return void
*/
public function report()
{
//
}
/**
* Render the exception into an HTTP response.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function render($request)
{
return response(...);
}
}
HTTP Exceptions:-
You have made some authorized pages. A user wants to access that authorized page, but we don’t need to access the page. So that we redirect to the error page(Unauthorized error – 401) using the abort helper function. You can write anywhere in your application.
abort(404);
The abort function contains two parameters. We pass the first parameter as the HTTP status code and the second parameter as the response text.
abort(403, 'Unauthorized action.');
Custom HTTP Error pages:
You want to customize the error page for the 404 HTTP status code, then create a 404.blade.php file inside the resource/views/errors directory. Laravel will run this page on all 404 errors generated by your application. You can also create a view named the HTTP status code. It will pass the abort function to the view as an exception variable.
{{ $exception->getMessage() }}
You may publish Laravel’s error page template with the vendor:publish Artisan command. After that, you may personalize them to fit your theme.
php artisan vendor:publish --tag=laravel-errors
I hope this article (how to deal with exceptions in Laravel) helps you to better understand the HTTP Exceptions and custom HTTP error pages in Laravel. For this article, I followed this link. If you have any questions, please leave a comment below and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,