Your cart is currently empty!
best way to handle front view in Laravel
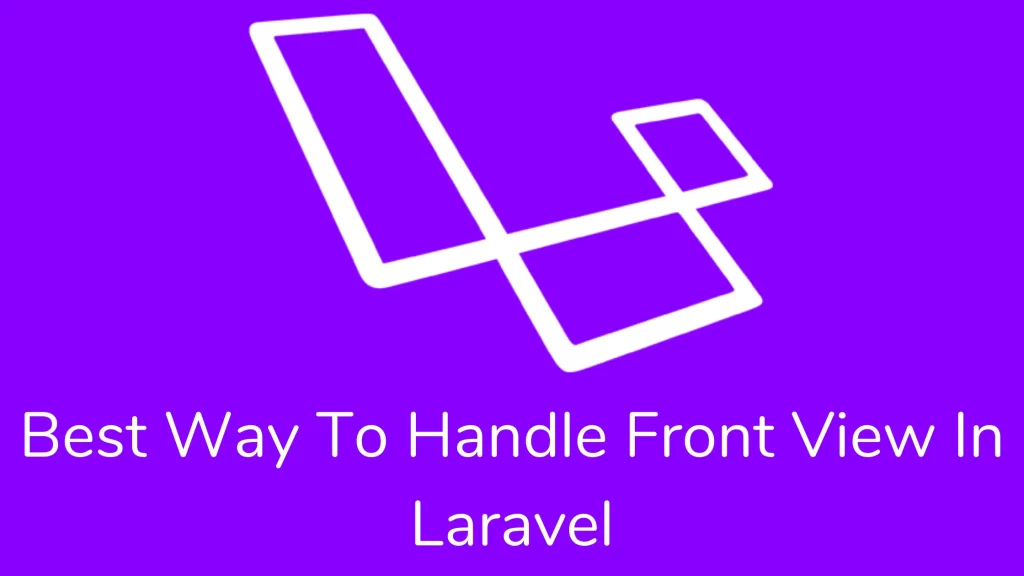
Hi guys,
We’ll look at the Best Way To Handle the Front View In Laravel today. Laravel generates the front view with the blade engine. The blade engine has a wide range of features that make it simple to adjust your view.
The blade is a simple, yet powerful templating engine provided by Laravel. You can also add PHP code inside the blade template. Blade views are stored in the resources/views directory, and the files are saved with the .blade.php extension.
Template Inheritance:-
You can easily inherit the view into another view. Suppose we create a master page layout that acts as a parent view.
<html>
<head>
<title>App Name - @yield('title')</title>
</head>
<body>
@section('sidebar')
This is the master sidebar.
@show
<div class="container">
@yield('content')
</div>
</body>
</html>
In the above code, we will see the two directives: @section, and @yield. The @section directives define a section of content. The @yield directive displays the content of the section.
Extending a Layout:
In the Child view, you use the @extend directive to extend the parent view. Child view injects the content into the layout section with the help of @section directives. The content of this section will be displayed on the layout using the @yield directive.
@extends('layouts.app')
@section('title', 'Page Title')
@section('sidebar')
@parent
<p>This is appended to the master sidebar.</p>
@endsection
@section('content')
<p>This is my body content.</p>
@endsection
The @parent directive will be replaced with the content of the layout when a view to rendered. @show directive will define and immediately yield the section.
@yield directive has a second parameter, which is the default value. It will render when the section being yielded is undefined.
@yield('content', View::make('view.name'))
Displaying Data:
You may pass the data to the view. You can access the variable to display value using curly braces.
Route::get('welcome', function () {
return view('welcome', ['name' => 'Samantha']);
});
Hello, {{$name}}
The blade allows you to call any PHP function, which echoes the view value. Also, you put any PHP code inside of a Blade echo statement.
The current UNIX timestamp is {{ time() }}.
By default, Laravel calls htmlspecialchars() function to prevent XSS attacks using {{ }} statement. You want to display data as captured.
Hello, {!! $name !!}
You wish to pass an array to your view but you want to access it in JSON format. For example,
<script>
var app = <?php echo json_encode($array); ?>;
</script>
Blade allows you to make JSON format using the @json directive, which accepts the same parameter as the json_encode function.
<script>
var app = @json($array);
var app = @json($array, JSON_PRETTY_PRINT);
</script>
Blade uses double encoded HTML entities. You want to disable it. You should call the Blade::withoutDoubleEncoding() method inside the boot method of AppServiceProvider.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Blade;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Blade::withoutDoubleEncoding();
}
}
Blade & Javascript Framework:
You may use the @ symbol to inform the blade engine to escape the expression and remove the @ symbol. Inside curly braces will be untouched and allow javascript to render it.
<h1>Laravel</h1>
Hello, @{{ name }}.
{{-- Blade --}}
@@json()
<!-- HTML output -->
@json()
The @verbatim Directive:
You are displaying JavaScript variables in much of your template. You may use the @verbatim directive. It helps you to not add too much @ symbol for each Blade echo statement.
@verbatim
Hello, {{ name }}.
@endverbatim
Control Structures:-
You may use conditional statements for displaying content using blade directives like @if, @elseif, @else, and @endif directives.
@if (count($records) === 1)
I have one record!
@elseif (count($records) > 1)
I have multiple records!
@else
I don't have any records!
@endif
The @isset and @empty directives may be used for shortcuts for their respective PHP functions.
@isset($records)
// $records is defined and is not null…
@endisset
@empty($records)
// $records is "empty"…
@endempty
The @auth and @guest directives may determine if the current user is authenticated or is a guest.
The @hasSection checks section has content. The @sectionMissing determines if the section does not have content.
Using the @production directive, you can run code in a production environment. Also, you can use the @env directive to run an application in a specific situation.
@env('staging')
// The application is running in "staging"…
@endenv
The loop variable-
The $loop variable available inside your loop gives you some effective bit of information.
@foreach ($users as $user)
@foreach ($user->posts as $post)
@if ($loop->parent->first)
This is the first iteration of the parent loop.
@endif
@endforeach
@endforeach
$loop variable contains a variety of other helpful properties. Check the table below:
Property | Description |
---|---|
$loop->index | The index of the current loop iteration (starts at 0). |
$loop->iteration | The current loop iteration (starts at 1). |
$loop->remaining | The iterations remaining in the loop. |
$loop->count | The total number of items in the array being iterated. |
$loop->first | Whether this is the first iteration through the loop. |
$loop->last | Whether this is the last iteration through the loop. |
$loop->even | Whether this is an even iteration through the loop. |
$loop->odd | Whether this is an odd iteration through the loop. |
$loop->depth | The nesting level of the current loop. |
$loop->parent | When in a nested loop, the parent’s loop variable. |
Comments in Blade:
You can also define comments on views like the below:
{{-- This comment will not be present in the rendered HTML --}}
The @php directive executes a block code on your view. We can define below:
@php
//
@endphp
The $once directive:
You are rendering components in a loop that time the Javascript library pushes into the body. It will load that library many times. Blade Engine introduces a $once directive that loads the library once as it renders it.
@once
@push('scripts')
<script>
// Your custom JavaScript...
</script>
@endpush
@endonce
Forms:-
CSRF Token:
To prevent Cross script Request Forgery(CSRF Token) the blade engine has introduced the @csrf directive. You can place it on the form. The blade engine automatically adds a hidden input token field.
<form method="POST" action="/profile">
@csrf
...
</form>
Method Form:
HTML Form does not support PUT, PATCH, or DELETE requests. You can add the @method directive inside the form. It will generate a hidden field as _method name.
<form action="/foo/bar" method="POST">
@method('PUT')
...
</form>
validation Form:
The @error directive checks whether validation error messages exist or not for a particular attribute.
<label for="title">Post Title</label>
<input id="title" type="text" class="@error('title') is-invalid @enderror">
@error('title')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
A Page contains multiple forms. You want to display error messages like below:
<label for="email">Email address</label>
<input id="email" type="email" class="@error('email', 'login') is-invalid @enderror">
@error('email', 'login')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
Including Subviews:-
The @include directive allows you to include a view in another view. All the data are available to the parent view. It is also accessible to included views, and you can pass extra data to be included view.
<div>
@include('shared.errors')
<form>
<!-- Form Contents -->
</form>
</div>
@include('view.name', ['some' => 'data'])
To check view exists or not then you use the @includeIf directive.
@includeIf('view.name', ['some' => 'data'])
After an expression evaluates to true, then you include the view.
@includeWhen($boolean, 'view.name', ['some' => 'data'])
An expression evaluates to false, child view can be included in the parent view.
@includeUnless($boolean, 'view.name', ['some' => 'data'])
To include the first view inside an array of views. You may use the @includeFirst directive.
@includeFirst(['custom.admin', 'admin'], ['some' => 'data'])
Rendering Views For Collections:-
You can add together loops and include them into one line with @each directive.
@each('view.name', $jobs, 'job')
The first argument is used for a view rendered for each element in an array. The second argument is collation or Array to be iterated. The third argument is the variable name which is used within view to render. You can also pass the fourth argument as a view. If the Array is empty, then the fourth argument is executed.
@each('view.name', $jobs, 'job', 'view.empty')
Stacks:-
You push value to the named stack, which can render somewhere else.
@push('scripts')
<script src="/example.js"></script>
@endpush
To render complete stack contents, you can check the following example
<head>
<!-- Head Contents -->
@stack('scripts')
</head>
You wish to prepend content onto the beginning of a stack.
@push('scripts')
This will be second...
@endpush
// Later...
@prepend('scripts')
This will be first...
@endprepend
Service Injection:-
The @inject directive is used to retrieve a service from a service container. The first argument is the name of the variable. The second argument is the class or interface name of the service.
@inject('metrics', 'App\Services\MetricsService')
<div>
Monthly Revenue: {{ $metrics->monthlyRevenue() }}.
</div>
Extending Blade:-
When the blade compiler encounters the custom directive, it will call the provided callback with the expression.
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Blade;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Blade::directive('datetime', function ($expression) {
return "<?php echo ($expression)->format('m/d/Y H:i'); ?>";
});
}
}
You can access the above directive like:
@directive($var)
Custom If Statements:
A Blade::if method allows you to define custom conditional directives using a closure. You may set this inside the boot method of AppServiceProvider.
use Illuminate\Support\Facades\Blade;
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Blade::if('cloud', function ($provider) {
return config('filesystems.default') === $provider;
});
}
After defining a conditional statement, you can easily use it on your templates.
@cloud('digitalocean')
// The application is using the digital ocean cloud provider
@elsecloud('aws')
// The application is using the AWS provider…
@else
// The application is not using the digital ocean or AWS environment…
@endcloud
@unlesscloud('aws')
// The application is not using the AWS environment…
@endcloud
I hope this article (best way to handle front view in Laravel) helps you to better understand the separate view for multiple use cases, conditional display views, and inheritance of another view. For this article, I followed this link. If you have questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,