Your cart is currently empty!
integrate the Razorpay payment gateway in laravel
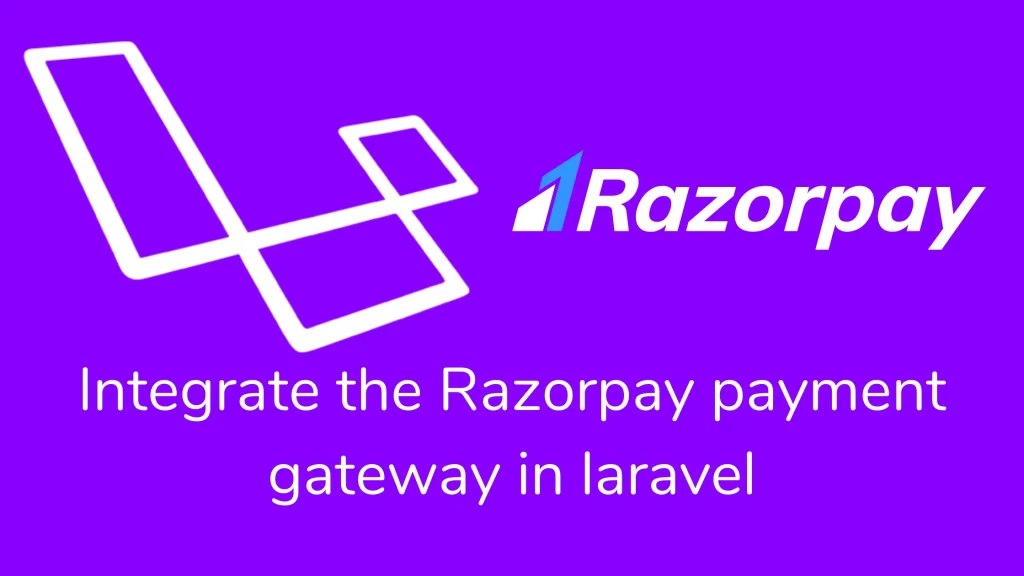
Hi guys,
If you want to get paid online from your application. You need to integrate a payment gateway into your application. In this article, we integrate the Razorpay payment gateway in laravel. So you will get passive income on your packet. If you want to create an account on Razorpay:- Click Here.
Some requirements need to integrate Razorpay in laravel:
- PHP version 5.3 or higher
- Create a Razorpay Account and Generate an API key.
Afterward, we are going through a step-by-step process to integrate the Razorpay payment gateway in laravel.
Step 1:- Install Razorpay PHP SDK in the project.
Run the below command on your composer:
composer require razorpay/razorpay
You can also download the latest razorpay-php.zip file from GitHub. The razorpay-php.zip is pre-compiled to include all dependencies. Unzip the razorpay-php.zip file inside your project and autoload the razorpay.php file in your application.
Step 2:- Integrate Orders API
Order initiation is a required step for every payment process. You can create orders and link them to payments. Once payment is confirmed, we denote the transaction as paid.
To initiate the order process, you use the create() function and pass an array of a parameter to it.
$orderData = [
'receipt' => 'rcptid_11',
'amount' => 39900, // 39900 rupees in paise
'currency' => 'INR',
'notes' => {"note_key": "Beam me up Scotty”},
'partial_payment' => true,
];
$razorpayOrder = $api->order->create($orderData);
The transaction amount should be multiple with 100(removing decimal points in the amount). The currency is a required field. Check your currency on a currency list and add them accordingly. Pass the receipt ID for creating an order. The notes are key-value pairs for extra information about the transaction. You can manage partial payment with a boolean value against partial_payment.
When the order is completed successfully, you can store the response against the order in your application. After payment is authorized, you can capture it to settle the amount in our bank account as per the settlement schedule.
Step 3:- Add Checkout Options
Add the below code inside the checkout page in your application.
<button id="rzp-button1">Pay with Razorpay</button>
<script src="https://checkout.razorpay.com/v1/checkout.js"></script>
<form name='razorpayform' action="verify.php" method="POST">
<input type="hidden" name="razorpay_payment_id" id="razorpay_payment_id">
<input type="hidden" name="razorpay_signature" id="razorpay_signature" >
</form>
<script>
// Checkout details as a json
var options = <?php echo $json?>;
/**
* The entire list of checkout fields is available at
* https://docs.razorpay.com/docs/checkout-form#checkout-fields
*/
options.handler = function (response){
document.getElementById('razorpay_payment_id').value = response.razorpay_payment_id;
document.getElementById('razorpay_signature').value = response.razorpay_signature;
document.razorpayform.submit();
};
// Boolean whether to show image inside a white frame. (default: true)
options.theme.image_padding = false;
var rzp = new Razorpay(options);
document.getElementById('rzp-button1').onclick = function(e){
rzp.open();
e.preventDefault();
}
</script>
Add the checkout parameters to the Razorpay object like the above example options.
options = {
"key" => keyId,
"amount" => amount,
"name" => "Acme Corp",
"description" => "Buy best price",
"image" => "https://cdn.razorpay.com/logos/FFATTsJeURNMxx_medium.png",
"prefill" => {
"name" => "Gaurav Kumar",
"email" => "[email protected]",
"contact" => "9999999999",
},
"notes" => {
"address" => "Hello World",
"merchant_order_id" => "12312321",
},
"theme" => {
"color" => "#99cc33"
},
"order_id" => razorpayOrderId,
};
The keyID is the API key generated in the Razorpay dashboard. The amount paid by the customer. We need currency for the payment. Enter your business name against the name field. It is a description of the purchase. You can see the image in the form.
You should add orderID from Orders API. The prefill is a key-value object which contains customer details. A set of key-value pairs are in the notes. The theme is a set of key-value pairs for change basis UI components.
Step 4- Store fields in the server
After they completed the transaction, Razorpay sent the following response. You can verify and store it in the database.
{
"razorpay_payment_id": "pay_29QQoUBi66xm2f",
"razorpay_order_id": "order_9A33XWu170gUtm",
"razorpay_signature": "9ef4dffbfd84f1318f6739a3ce19f9d85851857ae648f114332d8401e0949a3d"
}
Step 5:- Verify Payment Signature
You can confirm the authenticity of details returned to the checkout for successful payments. To verify the signature, you need to order_id (order_id pass as argument to Razorpay object), razorpay_payment_id (Return from checkout process), key_secret (generated from Razorpay dashboard)
Use the SHA256 algorithm, the razorpay_payment_id, and order_id to construct an HMAC hex digest.
generated_signature = hmac_sha256(order_id + "|" + razorpay_payment_id, secret);
if (generated_signature == razorpay_signature) {
payment is successful
}
You want to match signatures on the server side after returning from the checkout screen. The payment received is from an authentic source.
use Razorpay\Api\Api;
use Razorpay\Api\Errors\SignatureVerificationError;
$success = true;
$error = "Payment Failed";
if (empty($_POST['razorpay_payment_id']) === false)
{
$api = new Api($keyId, $keySecret);
try
{
// Please note that the razorpay order ID must
// come from a trusted source (session here, but
// could be database or something else)
$attributes = array(
'razorpay_order_id' => $_SESSION['order_id'],
'razorpay_payment_id' => $_POST['razorpay_payment_id'],
'razorpay_signature' => $_POST['razorpay_signature']
);
$api->utility->verifyPaymentSignature($attributes);
}
catch(SignatureVerificationError $e)
{
$success = false;
$error = 'Razorpay Error : ' . $e->getMessage();
}
}
if ($success === true)
{
$html = "<p>Your payment was successful</p>
<p>Payment ID: {$_POST['razorpay_payment_id']}</p>";
}
else
{
$html = "<p>Your payment failed</p>
<p>{$error}</p>";
}
echo $html;
We have successfully integrated the Razorpay payment gateway in laravel. We integrate one of the APIs in this article. For reference, you can refer to this document.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers