Your cart is currently empty!
Make custom Components in laravel in just 2 minutes
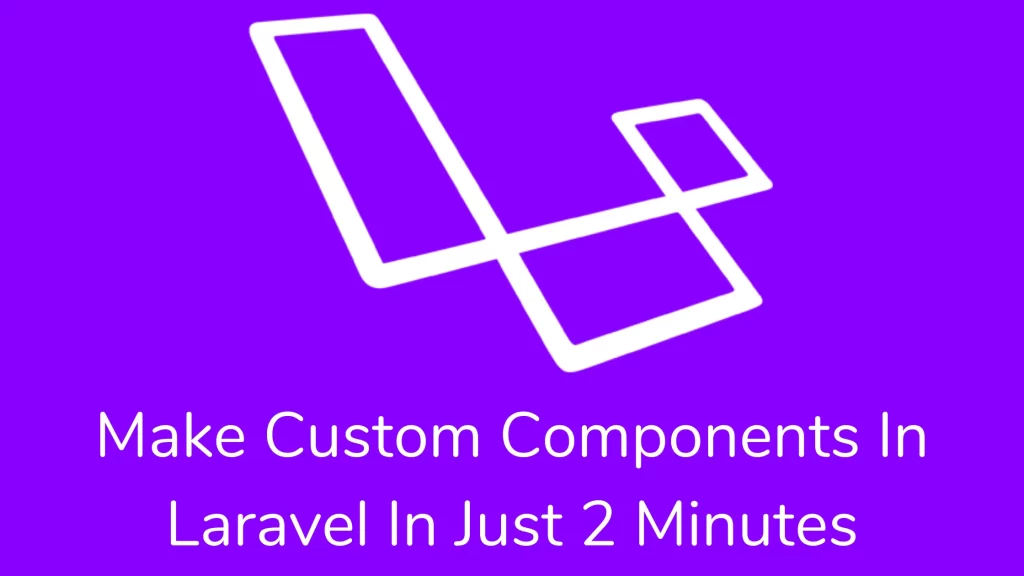
Hi Guys,
Today we’ll look into the creation of Custom Components in Laravel. We can pass data to the component, and it can manipulate the component based on the data. In perspective, a component is a tiny portion of a section. It’s the most effective technique to separate components in a view.
Components are the same as the layout or section. You can create components in two ways:-
- class-based component,
- anonymous component
class-based components:-
You may use the make Artisan command to create a new component, and it stores the components inside the App\View\Components directory. Run the below command to make a component:
php artisan make:component Alert
It also creates views inside the resources/views/components directory. You are utilizing your application. You should register your component class and HTML tag alias inside the boot method at your service provider.
use Illuminate\Support\Facades\Blade;
/**
* Bootstrap your package's services.
*/
public function boot()
{
Blade::component('package-alert', AlertComponent::class);
}
After registering your component, you can use an HTML tag alias in your application like below:
<x-package-alert/>
Blade components start with an x-string followed by a component name like the above. We nested deeper inside the components directory. You may use the ‘DOT’ operator for nesting the directory.
<x-inputs.button/>
Passing Data to components:
You can share data from the parent to the component via the attribute, which is a prefix with ‘:’ (COLON)
<x-alert type="error" :message="$message"/>
You should define the component’s data inside the constructor of the class. It will be automatically available to the component’s view. It is unnecessary to pass the data into the component view.
<?php
namespace App\View\Components;
use Illuminate\View\Component;
class Alert extends Component
{
/**
* The alert type.
*
* @var string
*/
public $type;
/**
* The alert message.
*
* @var string
*/
public $message;
/**
* Create the component instance.
*
* @param string $type
* @param string $message
* @return void
*/
public function __construct($type, $message)
{
$this->type = $type;
$this->message = $message;
}
/**
* Get the view/contents that represent the component.
*
* @return \Illuminate\View\View|\Closure|string
*/
public function render()
{
return view('components.alert');
}
}
The component view looks like below:
<div class="alert alert-{{ $type }}">
{{ $message }}
</div>
You must define arguments in the camel case. In HTML attributes, write an argument name like kebab-case.
public function __construct($alertType)
{
$this->alertType = $alertType;
}
<x-alert alert-type="danger" />
Public variables and methods are available in your component template.
public function isSelected($option)
{
return $option === $this->selected;
}
You may run this method from your component template.
<option {{ $isSelected($value) ? 'selected="selected"' : '' }} value="{{ $value }}">
{{ $label }}
</option>
You can access the component name, attributes, and slot inside the render method.
public function render()
{
return function (array $data) {
// $data['componentName'];
// $data['attributes'];
// $data['slot'];
return '<div>Component content</div>';
};
}
Your component needs some dependencies from the service container. You may mention it inside the constructor so that they automatically inject it.
use App\AlertCreator
/**
* Create the component instance.
*
* @param \App\AlertCreator $creator
* @param string $type
* @param string $message
* @return void
*/
public function __construct(AlertCreator $creator, $type, $message)
{
$this->creator = $creator;
$this->type = $type;
$this->message = $message;
}
You may pass additional attributes to a component like class attributes. It is automatically available to the component. You echo them.
<div {{ $attributes }}>
<!-- Component Content -->
</div>
Sometimes, you want to attribute value to attributes inside your components. The blade allows you to add them using the merge method.
<div {{ $attributes->merge(['class' => 'alert alert-'.$type]) }}>
{{ $message }}
</div>
The filter method accepts a Closure that returns true if you wish to keep the attribute.
{{ $attributes->filter(fn ($value, $key) => $key == 'foo') }}
You define public methods or properties on your component and access the component within your slot via the $component variable.
<x-alert>
<x-slot name="title">
{{ $component->formatAlert('Server Error') }}
</x-slot>
<strong>Whoops!</strong> Something went wrong!
</x-alert>
Inline Component Views:
You made a component that is tiny in code. It is not sufficient for the separate component class and component view. You can return component markup directly from the render method.
public function render()
{
return <<<'blade'
<div class="alert alert-danger">
{{ $slot }}
</div>
blade;
}
You can execute the following command to create an inline component:
php artisan make:component Alert --inline
Anonymous Components:-
To define an anonymous component, you need to create a component inside the resources/views/components directory. Do not create a component class.
<x-alert/>
You have made a nested component so you can use ‘.’ (DOT) character to deeper inside the components directory.
<x-inputs.button/>
You may specify the @props directive at the top of your component. So that attribute should be considered a data variable.
@props(['type' => 'info', 'message'])
<div {{ $attributes->merge(['class' => 'alert alert-'.$type]) }}>
{{ $message }}
</div>
I hope this article (Make Custom Components In Laravel) helps you better understand manipulate custom components. For this article, I followed this link. If you have questions, please leave a comment. I will respond as soon as possible.
Thank you for reading this article. Share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,