Your cart is currently empty!
How To manage collection data in laravel?
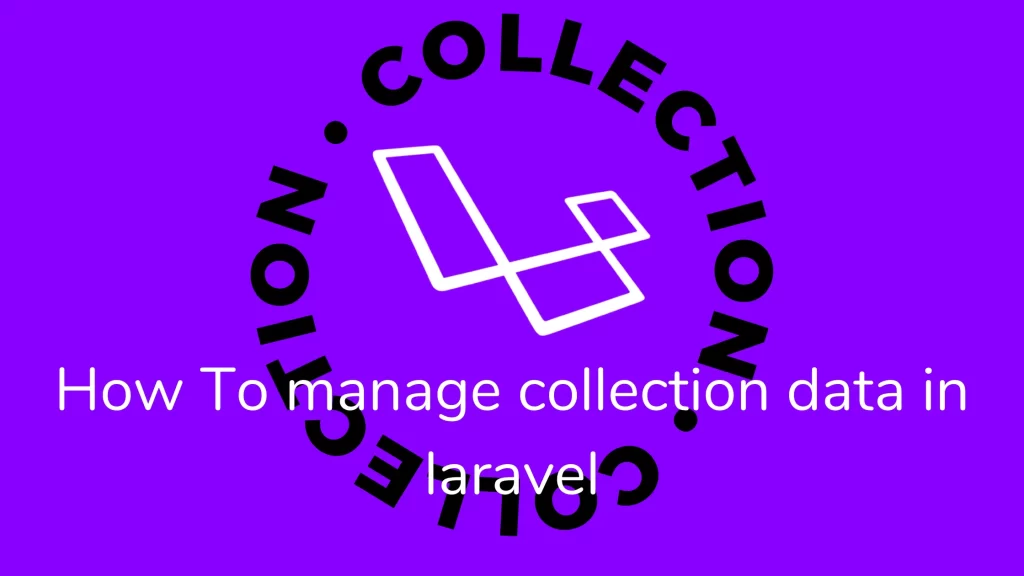
Hi guys,
The Collection class provides a convenient wrapper for working on an array of data. we are to manage the collection in laravel. Below is an example of a collect helper that creates a new collection of instances, processes the upper case on the element, and removes the nullable element.
$collection = collect(['taylor', 'abigail', null])->map(function ($name) {
return strtoupper($name);
})
->reject(function ($name) {
return empty($name);
});
Creating Collection-
The collect helper returns a new collection instance for the given array. Every Eloquent query returns collection instances. Afterward, we will manage the collection data in laravel.
$collection = collect([1,2,4]);
Extending Collections-
You can add extra methods to the Collection class at run time and write this to the service provider. The below code is an example of extending collection.
use Illuminate\Support\Collection;
use Illuminate\Support\Str;
Collection::macro('toUpper', function () {
return $this->map(function ($value) {
return Str::upper($value);
});
});
$collection = collect(['first', 'second']);
$upper = $collection->toUpper();
// ['FIRST', 'SECOND']
Available Methods:-
We can use methods to manage collection data in laravel. Every method returns a new Collection instance. you can preserve original data.
all() – return the underlying array
collect([1, 2, 3])->all();
// [1, 2, 3]
avaerage()/ avg() – returns the average value of a given key
$average = collect([['foo' => 10], ['foo' => 10], ['foo' => 20], ['foo' => 40]])->avg('foo');
//20
$average = collect([1, 1, 2, 4])->avg();
//2
chunk() – breaks the collection into multiple collections of a given size. You are working on grid view. Here, we use this.
$collection = collect([1, 2, 3, 4, 5, 6, 7]);
$chunks = $collection->chunk(4);
$chunks->toArray();
collapse() – merge all collections of an array into one collection.
$collection = collect([[1, 2, 3], [4, 5, 6], [7, 8, 9]]);
$collapsed = $collection->collapse();
$collapsed->all();
combine() – incorporate the values of the collection as keys along with values of other collections.
$collection = collect(['name', 'age']);
$combined = $collection->combine(['George', 29]);
$combined->all();
collect() – return a new collection instance with items currently in the collection. The collect() is useful for converting lazy collection to standard collection.
$collectionA = collect([1, 2, 3]);
$collectionB = $collectionA->collect();
$collectionB->all();
$lazyCollection = LazyCollection::make(function () {
yield 1;
yield 2;
yield 3;
});
$collection = $lazyCollection->collect();
get_class($collection);
// 'Illuminate\Support\Collection'
$collection->all();
concat() – appends the given array or collection values.
$collection = collect(['John Doe']);
$concatenated = $collection->concat(['Jane Doe'])->concat(['name' => 'Johnny Doe']);
$concatenated->all();
contains() – check whether the collection contains an item. You can key-value pair to the contains() method.
$collection = collect(['name' => 'Desk', 'price' => 100]);
$collection->contains('Desk');
// true
$collection->contains('New York');
$collection = collect([
['product' => 'Desk', 'price' => 200],
['product' => 'Chair', 'price' => 100],
]);
$collection->contains('product', 'Bookcase');
containStrict() – same functionality as contain() method. Also, it compared strictly.
count() – return the total number of items in the collection. You can pass the callback function to count all items.
$collection = collect([1, 2, 3, 4]);
$collection->count();
countBy() – counts of items that occurred multiple times.
$collection = collect([1, 2, 2, 2, 3]);
$counted = $collection->countBy();
$counted->all();
$collection = collect(['[email protected]', '[email protected]', '[email protected]']);
$counted = $collection->countBy(function ($email) {
return substr(strrchr($email, "@"), 1);
});
$counted->all();
crossJoin() – return a cartesian product to all possible combinations.
$collection = collect([1, 2]);
$matrix = $collection->crossJoin(['a', 'b'], ['I', 'II']);
$matrix->all();
/*
[
[1, 'a', 'I'],
[1, 'a', 'II'],
[1, 'b', 'I'],
[1, 'b', 'II'],
[2, 'a', 'I'],
[2, 'a', 'II'],
[2, 'b', 'I'],
[2, 'b', 'II'],
]
*/
dd() – dumps all collections and stops the execution.
$collection = collect(['John Doe', 'Jane Doe']);
$collection->dd();
diff() – compare the collections and returns not a common item of the first collection.
$collection = collect([1, 2, 3, 4, 5]);
$diff = $collection->diff([2, 4, 6, 8]);
$diff->all();
// [1, 3, 5]
diffAssoc() – We are comparing the collection with another collection. It will return the original key-value pair, but not from another collection.
$collection = collect([
'color' => 'orange',
'type' => 'fruit',
'remain' => 6,
]);
$diff = $collection->diffAssoc([
'color' => 'yellow',
'type' => 'fruit',
'remain' => 3,
'used' => 6,
]);
$diff->all();
// ['color' => 'orange', 'remain' => 6]
diffKeys() – we are comparing the collection with another collection based on keys. It will return the key-value pair from the first collection.
$collection = collect([
'one' => 10,
'two' => 20,
'three' => 30,
'four' => 40,
'five' => 50,
]);
$diff = $collection->diffKeys([
'two' => 2,
'four' => 4,
'six' => 6,
'eight' => 8,
]);
$diff->all();
// ['one' => 10, 'three' => 30, 'five' => 50]
dump() – dump the collections items
$collection = collect(['John Doe', 'Jane Doe']);
$collection->dump();
duplicates() – return duplicates values from collection. You can pass the key to the duplicates method for an associative array.
$collection = collect(['a', 'b', 'a', 'c', 'b']);
$collection->duplicates();
$employees = collect([
['email' => '[email protected]', 'position' => 'Developer'],
['email' => '[email protected]', 'position' => 'Designer'],
['email' => '[email protected]', 'position' => 'Developer'],
])
$employees->duplicates('position');
duplicatesStrict() – It is the same signature as the duplicate() method for comparing data strictly.
each() – iterates items from the collection
$collection->each(function ($item, $key) {
if (/* some condition */) {
return false;
}
});
eachSpread() – iterates over the collection items, and passes each nested item value.
$collection = collect([['John Doe', 35], ['Jane Doe', 33]]);
$collection->eachSpread(function ($name, $age) {
//
});
every() – verify all elements of the collection satisfy the condition. If the collection is empty, every time, it will return true.
collect([1, 2, 3, 4])->every(function ($value, $key) {
return $value > 2;
});
except() – return all items of collection except those specified keys.
$collection = collect(['product_id' => 1, 'price' => 100, 'discount' => false]);
$filtered = $collection->except(['price', 'discount']);
$filtered->all();
filter() – return items that satisfy the conditions from the collection.
$collection = collect([1, 2, 3, 4]);
$filtered = $collection->filter(function ($value, $key) {
return $value > 2;
});
$filtered->all();
first() – return the first item from a collection that satisfies the condition.
collect([1, 2, 3, 4])->first(function ($value, $key) {
return $value > 2;
});
firstWhere() – return the first element from the collection with a given key-value pair.
$collection = collect([
['name' => 'Regena', 'age' => null],
['name' => 'Linda', 'age' => 14],
['name' => 'Diego', 'age' => 23],
['name' => 'Linda', 'age' => 84],
]);
$collection->firstWhere('name', 'Linda');
flatMap() – iterates through the collection and passes each value to the callback. The callback is free to change the item and return it.
$collection = collect([
['name' => 'Sally'],
['school' => 'Arkansas'],
['age' => 28]
]);
$flattened = $collection->flatMap(function ($values) {
return array_map('strtoupper', $values);
});
$flattened->all();
flatten() – convert multi-dimensional to a single dimension
$collection = collect(['name' => 'taylor', 'languages' => ['php', 'javascript']]);
$flattened = $collection->flatten();
$flattened->all();
flip() – swaps the keys with corresponding values.
$collection = collect(['name' => 'taylor', 'framework' => 'laravel']);
$flipped = $collection->flip();
$flipped->all();
forget() – remove the item from the collection.
$collection = collect(['name' => 'taylor', 'framework' => 'laravel']);
$collection->forget('name');
$collection->all();
forPage() – return a new collection containing the items. We can pass two arguments. The first argument is page number, and the second parameter is item per page.
$collection = collect([1, 2, 3, 4, 5, 6, 7, 8, 9]);
$chunk = $collection->forPage(2, 3);
$chunk->all();
get() – return item at a given key
$collection = collect(['name' => 'taylor', 'framework' => 'laravel']);
$value = $collection->get('name');
groupBy() – groups of collection items from the given key.
$collection = collect([
['account_id' => 'account-x10', 'product' => 'Chair'],
['account_id' => 'account-x10', 'product' => 'Bookcase'],
['account_id' => 'account-x11', 'product' => 'Desk'],
]);
$grouped = $collection->groupBy('account_id');
$grouped->toArray();
We may pass multiple grouping criteria as an array. Each array element will be applied to the corresponding level within a multi-dimensional array:
$data = new Collection([
10 => ['user' => 1, 'skill' => 1, 'roles' => ['Role_1', 'Role_3']],
20 => ['user' => 2, 'skill' => 1, 'roles' => ['Role_1', 'Role_2']],
30 => ['user' => 3, 'skill' => 2, 'roles' => ['Role_1']],
40 => ['user' => 4, 'skill' => 2, 'roles' => ['Role_2']],
]);
$result = $data->groupBy([
'skill', function ($item) {
return $item['roles'];
},
], $preserveKeys = true);
has() – if a given key exists in the collection.
$collection = collect(['account_id' => 1, 'product' => 'Desk', 'amount' => 5]);
$collection->has('product');
implode() – join the items in a collection. You should pass the key of attributes you wish to join and the delimiter string.
$collection = collect([
['account_id' => 1, 'product' => 'Desk'],
['account_id' => 2, 'product' => 'Chair'],
]);
$collection->implode('product', ', ');
// Desk, Chair
intersect() – removes any values from the original collection. Those are not present in the given array.
$collection = collect(['Desk', 'Sofa', 'Chair']);
$intersect = $collection->intersect(['Desk', 'Chair', 'Bookcase']);
$intersect->all();
intersectByKeys() – removes any keys from a collection that does not exist in the array.
$collection = collect([
'serial' => 'UX301', 'type' => 'screen', 'year' => 2009,
]);
$intersect = $collection->intersectByKeys([
'reference' => 'UX404', 'type' => 'tab', 'year' => 2011,
]);
$intersect->all();
isEmpty() – checks whether the collection is empty or not.
isNotEmpty() – checks the collection is not empty.
collect([])->isEmpty();
collect([])->isEmpty();
join() – join the collection’s values with a string.
collect(['a', 'b', 'c'])->join(', ', ', and ');
keyBy() – multiple items have the same key.
$collection = collect([
['product_id' => 'prod-100', 'name' => 'Desk'],
['product_id' => 'prod-200', 'name' => 'Chair'],
]);
$keyed = $collection->keyBy('product_id');
$keyed->all();
/*
[
'prod-100' => ['product_id' => 'prod-100', 'name' => 'Desk'],
'prod-200' => ['product_id' => 'prod-200', 'name' => 'Chair'],
]
*/
keys() – return all collection keys
$collection = collect([
'prod-100' => ['product_id' => 'prod-100', 'name' => 'Desk'],
'prod-200' => ['product_id' => 'prod-200', 'name' => 'Chair'],
]);
$keys = $collection->keys();
$keys->all();
// ['prod-100', 'prod-200']
last() – return last element from collection.
collect([1, 2, 3, 4])->last(function ($value, $key) {
return $value < 3;
});
macro() – allows you to add a method to the Collection class at runtime.
make() – creates a new collection instance.
map() – loop through the collection. it passed each value to the given callback.
$collection = collect([1, 2, 3, 4, 5]);
$multiplied = $collection->map(function ($item, $key) {
return $item * 2;
});
$multiplied->all();
mapInto() – iterates over the collection, creating a new class instance.
class Currency
{
/**
* Create a new currency instance.
*
* @param string $code
* @return void
*/
function __construct(string $code)
{
$this->code = $code;
}
}
$collection = collect(['USD', 'EUR', 'GBP']);
$currencies = $collection->mapInto(Currency::class);
$currencies->all();
mapSpread() – iterates over the collection’s items passing each nested item value into a callback.
$collection = collect([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]);
$chunks = $collection->chunk(2);
$sequence = $chunks->mapSpread(function ($even, $odd) {
return $even + $odd;
});
$sequence->all();
mapToGroups()– return an associative array containing a single key-value pair
$collection = collect([
[
'name' => 'John Doe',
'department' => 'Sales',
],
[
'name' => 'Jane Doe',
'department' => 'Sales',
],
[
'name' => 'Johnny Doe',
'department' => 'Marketing',
]
]);
$grouped = $collection->mapToGroups(function ($item, $key) {
return [$item['department'] => $item['name']];
});
$grouped->toArray();
/*
[
'Sales' => ['John Doe', 'Jane Doe'],
'Marketing' => ['Johnny Doe'],
]
*/
$grouped->get('Sales')->all();
// ['John Doe', 'Jane Doe']
mapWithKeys() – iterates through the collection and passes each value to a given callback.
$collection = collect([
[
'name' => 'John',
'department' => 'Sales',
'email' => '[email protected]',
],
[
'name' => 'Jane',
'department' => 'Marketing',
'email' => '[email protected]',
]
]);
$keyed = $collection->mapWithKeys(function ($item) {
return [$item['email'] => $item['name']];
});
$keyed->all();
max() – return the maximum value of a given key.
$max = collect([1, 2, 3, 4, 5])->max();
median() – return the median value of given key
$median = collect([1, 1, 2, 4])->median();
merge() – merge the two array or collection.
$collection = collect(['product_id' => 1, 'price' => 100]);
$merged = $collection->merge(['price' => 200, 'discount' => false]);
$merged->all();
mergeRecursive() – A string key from a collection matches a string key from another collection and values for these keys are merged into an array.
$collection = collect(['product_id' => 1, 'price' => 100]);
$merged = $collection->mergeRecursive(['product_id' => 2, 'price' => 200, 'discount' => false]);
$merged->all();
min() – minimum value of a given key.
$min = collect([['foo' => 10], ['foo' => 20]])->min('foo');
// 10
mode() – return the mode value of a given key.
$mode = collect([['foo' => 10], ['foo' => 10], ['foo' => 20], ['foo' => 40]])->mode('foo');
// [10]
nth() – creates a new collection consisting of every nth element
$collection = collect(['a', 'b', 'c', 'd', 'e', 'f']);
$collection->nth(4);
only() – returns the items from a collection with a specified key.
$collection = collect(['product_id' => 1, 'name' => 'Desk', 'price' => 100, 'discount' => false]);
$filtered = $collection->only(['product_id', 'name']);
$filtered->all();
// ['product_id' => 1, 'name' => 'Desk']
pad() – fill the array with the given value until an array reaches the specified size.
$collection = collect(['A', 'B', 'C']);
$filtered = $collection->pad(5, 0);
$filtered->all();
// ['A', 'B', 'C', 0, 0]
partition() – separate elements that pass a given truth test from those that do not.
$collection = collect([1, 2, 3, 4, 5, 6]);
list($underThree, $equalOrAboveThree) = $collection->partition(function ($i) {
return $i < 3;
});
$underThree->all();
// [1, 2]
$equalOrAboveThree->all();
// [3, 4, 5, 6]
pipe() – passes the collection to given callback
$collection = collect([1, 2, 3]);
$piped = $collection->pipe(function ($collection) {
return $collection->sum();
});
pluck() – retrieves all the values for given key.
$collection = collect([
['product_id' => 'prod-100', 'name' => 'Desk'],
['product_id' => 'prod-200', 'name' => 'Chair'],
]);
$plucked = $collection->pluck('name');
$plucked->all();
// ['Desk', 'Chair']
pop() – remove last item from collection
$collection = collect([1, 2, 3, 4, 5]);
$collection->pop();
prepend() – adds item at beginning of the collection.
$collection = collect([1, 2, 3, 4, 5]);
$collection->prepend(0);
$collection->all();
// [0, 1, 2, 3, 4, 5]
pull() – removes an item from the collection using its key
$collection = collect(['product_id' => 'prod-100', 'name' => 'Desk']);
$collection->pull('name');
push() – appends an item to the end of the collection
$collection = collect([1, 2, 3, 4]);
$collection->push(5);
$collection->all();
// [1, 2, 3, 4, 5]
put() – sets the given key and value in the collection
$collection = collect(['product_id' => 1, 'name' => 'Desk']);
$collection->put('price', 100);
$collection->all();
random() – returns a random item from collection
$collection = collect([1, 2, 3, 4, 5]);
$collection->random();
reduce() – reduce the collection into single value
$collection = collect([1, 2, 3]);
$total = $collection->reduce(function ($carry, $item) {
return $carry + $item;
});
// 6
reject() – If the condition is true, we remove the item from the collection.
$collection = collect([1, 2, 3, 4]);
$filtered = $collection->reject(function ($value, $key) {
return $value > 2;
});
replace() – overwrite items in the collection that match numeric keys
$collection = collect(['Taylor', 'Abigail', 'James']);
$replaced = $collection->replace([1 => 'Victoria', 3 => 'Finn']);
$replaced->all();
replaceRecursive() will recur into an array and apply the same replacement process to the inner values.
$collection = collect(['Taylor', 'Abigail', ['James', 'Victoria', 'Finn']]);
$replaced = $collection->replaceRecursive(['Charlie', 2 => [1 => 'King']]);
$replaced->all();
reverse() – reverse the order of collection items.
$collection = collect(['a', 'b', 'c', 'd', 'e']);
$reversed = $collection->reverse();
$reversed->all();
search() – return the key of the collection if they found it. Otherwise, it returns false.
$collection = collect([2, 4, 6, 8]);
$collection->search(4);
shift() – remove the first item from the collection.
$collection = collect([1, 2, 3, 4, 5]);
$collection->shift();
shuffle() – randomly shuffle the items in the collection.
$collection = collect([1, 2, 3, 4, 5]);
$shuffled = $collection->shuffle();
$shuffled->all();
skip() – return a new collection, without the first given amount of items.
$collection = collect([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]);
$collection = $collection->skip(4);
$collection->all();
skipUntil() – skip items until the callback function returns true.
collection = collect([1, 2, 3, 4]);
$subset = $collection->skipUntil(function ($item) {
return $item >= 3;
});
$subset->all();
skipWhile() – skip items while given callback returns are true and return remaining items in the collection.
$collection = collect([1, 2, 3, 4]);
$subset = $collection->skipWhile(function ($item) {
return $item <= 3;
});
$subset->all();
slice() – return a slice of collection starting at the index.
$collection = collect([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]);
$slice = $collection->slice(4);
$slice->all();
some() – same as contains method
sort() – sorts the collection. You use the values method to reset the keys to consecutively numbered indexes.
$collection = collect([5, 3, 1, 2, 4]);
$sorted = $collection->sort();
$sorted->values()->all();
sortBy() – sorts the collection by given key
$collection = collect([
['name' => 'Desk', 'price' => 200],
['name' => 'Chair', 'price' => 100],
['name' => 'Bookcase', 'price' => 150],
]);
$sorted = $collection->sortBy('price');
$sorted->values()->all();
sortByDesc() – sort collection in the opposite order.
sortDesc() – sort the collection in the opposite order.
$collection = collect([5, 3, 1, 2, 4]);
$sorted = $collection->sortDesc();
$sorted->values()->all();
sortKeys() – sort the collection by the keys of the underlying associative array.
$collection = collect([
'id' => 22345,
'first' => 'John',
'last' => 'Doe',
]);
$sorted = $collection->sortKeys();
$sorted->all();
sortKeysDesc() – sort the collection in opposite order.
splice() – removes a slice of items starting at specified index
$collection = collect([1, 2, 3, 4, 5]);
$chunk = $collection->splice(2);
$chunk->all();
split() – breaks a collection into given number groups
$collection = collect([1, 2, 3, 4, 5]);
$groups = $collection->split(3);
$groups->toArray();
sum() – returns a sum of all items in the collection.
collect([1, 2, 3, 4, 5])->sum();
take() – return a new collection with the specified number of items
$collection = collect([0, 1, 2, 3, 4, 5]);
$chunk = $collection->take(3);
$chunk->all();
takeUtil() – return items in a collection until the given callback return is true.
$collection = collect([1, 2, 3, 4])
$subset = $collection->takeUntil(function ($item) {
return $item >= 3;
});
$subset->all();
takeWhile() – return items in a collection until the given callback returns false.
$collection = collect([1, 2, 3, 4]);
$subset = $collection->takeWhile(function ($item) {
return $item < 3;
});
$subset->all();
tap() – passes the collection to the given callback and does something with the items while not affecting the collection itself.
collect([2, 4, 3, 1, 5])
->sort()
->tap(function ($collection) {
Log::debug('Values after sorting', $collection->values()->toArray());
})->shift();
times() – creates a new collection by invoking the callback a given amount of times
$collection = Collection::times(10, function ($number) {
return $number * 9;
});
$collection->all();
toArray() – converts the collection into a plain array.
$collection = collect(['name' => 'Desk', 'price' => 200]);
$collection->toArray();
toJson() – convert the collection to a JSON serialized string.
$collection = collect(['name' => 'Desk', 'price' => 200]);
$collection->toJson();
// '{"name":"Desk", "price":200}'
transform() – iterates over the collection and calls the callback with each item in the collection.
$collection = collect([1, 2, 3, 4, 5]);
$collection->transform(function ($item, $key) {
return $item * 2;
});
$collection->all();
union() – adds given array to the collection. If the given array contains keys that are already in the original collection, the original collection’s values will be preferred:
$collection = collect([1 => ['a'], 2 => ['b']]);
$union = $collection->union([3 => ['c'], 1 => ['b']]);
$union->all();
unique() – returns all of the unique items from the collection.
$collection = collect([1, 1, 2, 2, 3, 4, 2]);
$unique = $collection->unique();
$unique->values()->all();
uniqueStrict() – same signature as a unique method but it compares strictly.
unless() – execute the given callback unless the first argument handed over to the method evaluates true.
$collection = collect([1, 2, 3]);
$collection->unless(true, function ($collection) {
return $collection->push(4);
});
$collection->unless(false, function ($collection) {
return $collection->push(5);
});
$collection->all();
unwrap() – return the collections’ underlying items from the value when applicable.
Collection::unwrap(collect('John Doe'));
// ['John Doe']
Collection::unwrap(['John Doe']);
// ['John Doe'].
Collection::unwrap('John Doe');
// 'John Doe'
values() – return a new collection with keys reset to consecutive integers.
$collection = collect([
10 => ['product' => 'Desk', 'price' => 200],
11 => ['product' => 'Desk', 'price' => 200],
]);
$values = $collection->values();
$values->all();
when() – execute the given callback the first argument evaluates to true.
$collection = collect([1, 2, 3]);
$collection->when(true, function ($collection) {
return $collection->push(4);
});
$collection->when(false, function ($collection) {
return $collection->push(5);
});
$collection->all();
whenEmpty() – execute the given callback when the collection is empty.
$collection = collect();
$collection->whenEmpty(function ($collection) {
return $collection->push('adam');
});
$collection->all();
// ['adam']
whenNotEmpty() – execute given callback when the collection is not empty.
$collection = collect(['michael', 'tom']);
$collection->whenNotEmpty(function ($collection) {
return $collection->push('adam');
});
$collection->all();
// ['michael', 'tom', 'adam']
$collection = collect();
$collection->whenNotEmpty(function ($collection) {
return $collection->push('adam');
});
$collection->all();
// []
where() – filters the collection by a given key-value pair.
$collection = collect([
['product' => 'Desk', 'price' => 200],
['product' => 'Chair', 'price' => 100],
['product' => 'Bookcase', 'price' => 150],
['product' => 'Door', 'price' => 100],
]);
$filtered = $collection->where('price', 100);
$filtered->all();
whereStrict() – the same signature as the where method, but it is compared strictly.
whereBetween() – filters the collection within a given range
$collection = collect([
['product' => 'Desk', 'price' => 200],
['product' => 'Chair', 'price' => 80],
['product' => 'Bookcase', 'price' => 150],
['product' => 'Pencil', 'price' => 30],
['product' => 'Door', 'price' => 100],
]);
$filtered = $collection->whereBetween('price', [100, 200]);
$filtered->all();
whereIn() – filters collection by a key value contained within a given array
$collection = collect([
['product' => 'Desk', 'price' => 200],
['product' => 'Chair', 'price' => 100],
['product' => 'Bookcase', 'price' => 150],
['product' => 'Door', 'price' => 100],
]);
$filtered = $collection->whereIn('price', [150, 200]);
$filtered->all();
whereInstanceOf() – filters the collection by a given class type.
use App\User;
use App\Post;
$collection = collect([
new User,
new User,
new Post,
]);
$filtered = $collection->whereInstanceOf(User::class);
$filtered->all();
whereNotBetween() – filter the collection not within a given range.
$collection = collect([
['product' => 'Desk', 'price' => 200],
['product' => 'Chair', 'price' => 80],
['product' => 'Bookcase', 'price' => 150],
['product' => 'Pencil', 'price' => 30],
['product' => 'Door', 'price' => 100],
]);
$filtered = $collection->whereNotBetween('price', [100, 200]);
$filtered->all();
whereNotIn() – filters the collection with a key value not contained within a given array.
$collection = collect([
['product' => 'Desk', 'price' => 200],
['product' => 'Chair', 'price' => 100],
['product' => 'Bookcase', 'price' => 150],
['product' => 'Door', 'price' => 100],
]);
$filtered = $collection->whereNotIn('price', [150, 200]);
$filtered->all();
whereNotInStrict() – same signature as whereNotIn method but it compares strictly.
whereNotNull() – filters items where the given key is not null.
$collection = collect([
['name' => 'Desk'],
['name' => null],
['name' => 'Bookcase'],
]);
$filtered = $collection->whereNotNull('name');
$filtered->all();
whereNull() – filters items where the given key is a null
$collection = collect([
['name' => 'Desk'],
['name' => null],
['name' => 'Bookcase'],
]);
$filtered = $collection->whereNull('name');
$filtered->all();
wrap() – wraps the given value in a collection
$collection = Collection::wrap('John Doe');
$collection->all();
// ['John Doe']
zip() – merges together the values of the given array with values.
$collection = collect(['Chair', 'Desk']);
$zipped = $collection->zip([100, 200]);
$zipped->all();
Lazy Collections:-
LazyCollection class allows you to work with low memory usage on large datasets. The Lazy collection may be kept only a small part of the file in memory at a given time.
use App\LogEntry;
use Illuminate\Support\LazyCollection;
LazyCollection::make(function () {
$handle = fopen('log.txt', 'r');
while (($line = fgets($handle)) !== false) {
yield $line;
}
})->chunk(4)->map(function ($lines) {
return LogEntry::fromLines($lines);
})->each(function (LogEntry $logEntry) {
// Process the log entry...
});
The query builder’s cursor method returns a LazyCollection instance. It reduces memory usage.
$users = App\User::cursor()->filter(function ($user) {
return $user->id > 500;
});
foreach ($users as $user) {
echo $user->id;
}
Creating Lazy Collection-
you can create a lazy collection instance using the make method.
use Illuminate\Support\LazyCollection;
LazyCollection::make(function () {
$handle = fopen('log.txt', 'r');
while (($line = fgets($handle)) !== false) {
yield $line;
}
});
Lazy Collection Methods-
tapEach() –
you can call the given callback as the items are being pulled out of the list individually.
$lazyCollection = LazyCollection::times(INF)->tapEach(function ($value) {
dump($value);
});
// Nothing displayed so far
$array = $lazyCollection->take(3)->all();
// 1
// 2
// 3
remember() – return a new lazy collection that will recognize any values. We have already enumerated it.
$users = User::cursor()->remember();
// No query executed yet
$users->take(5)->all();
// The query was executing, and the first 5 users were hydrating from the database
$users->take(20)->all();
// First 5 users come from the collection's cache. The rest are hydrating from the database.
I hope that this post (How to manage collection data in laravel) has clarified how to build and manipulate collection data. I used this site to write this post. If you have any questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers