Your cart is currently empty!
how to validate user Input with Laravel validation (Part – I)
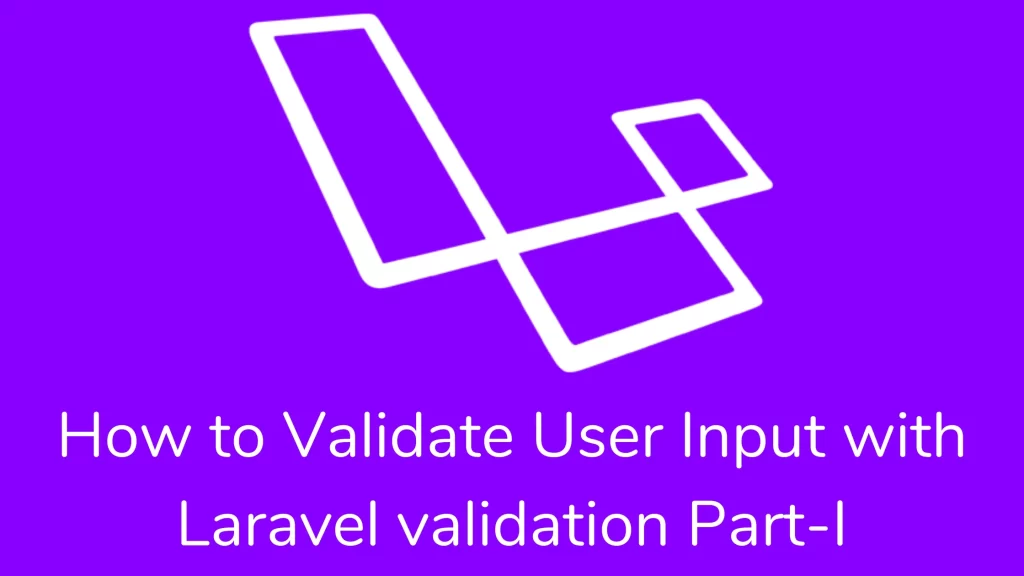
Hi guys,
Today we are learning how to validate user Input with Laravel. Laravel allows you to handle incoming data from requests. If validation fails, it throws an error message. For this topic, I will create two articles. In the first article, we are learning Form Request validation and displaying Validation Errors. The second article will study creating manual validators, working with error messages, and Custom Validation Rules. So let’s begin.
How to write validation logic:-
If the validation rules pass, then your code will keep executing normally. Otherwise, it gives an error response sent back to the user. For that, we are using the validate() method from the Illuminate\Http\Request object. So it looks like below:
public function store(Request $request)
{
$validatedData = $request->validate([
'title' => 'required|unique:posts|max:255',
'body' => 'required',
]);
// The blog post is valid
}
We are passing an array of rules to validate the method. If the validation fails, it halts the execution and sets back a proper response to the user. Otherwise, the process will continue to execute normally. You can also pass many validation rules for the particular entity as an array or single ‘|’ delimiter string.
$validatedData = $request->validate([
'title' => ['required', 'unique:posts', 'max:255'],
'body' => ['required'],
]);
You can use the validateWithBag method to validate a request and store any error messages with a named error bag.
$validatedData = $request->validateWithBag('post', [
'title' => ['required', 'unique:posts', 'max:255'],
'body' => ['required'],
]);
Stopping on first validation failure:
You want to stop running validation rules on an attribute. You can use the ‘bail’ keyword. In the below example, the blog’s title must be unique in our database. If it fails, it can’t check max character validation. It will validate rules in the order we provide them.
$request->validate([
'title' => 'bail|required|unique:posts|max:255',
'body' => 'required',
]);
Nested Attribute:
If your request contains a Nested parameter, you may specify them in your validation rules using “DOT” notation.
$request->validate([
'title' => 'required|unique:posts|max:255',
'author.name' => 'required',
'author.description' => 'required',
]);
Displaying Validation Errors:-
We did not explicitly bind the error message to the view. Because Laravel will check errors in session data, and it automatically binds to view. It wrapped the $errors variable to view. The web middleware group provides ShareErrorsFromSession middleware. So that is why it is always available to view. After validation fails, the Response will redirect to its previous view with an error message, and we are displaying them like below:
<h1>Create Post</h1>
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
The @error directive:
You may also use the @error directive to check an attribute that throws an error. You can display an error message on view.
<label for="title">Post Title</label>
<input id="title" type="text" class="@error('title') is-invalid @enderror">
@error('title')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
Optional Fields:
You will often need to mark optional fields as nullable if you do not want the validator to consider null values as invalid.
$request->validate([
'title' => 'required|unique:posts|max:255',
'body' => 'required',
'publish_at' => 'nullable|date',
]);
In the AJAX request, Laravel will generate a JSON response with all validation errors along with the 422 HTTP status code.
Form Request Validation:-
You want to create a custom request class that contains validation rules. It simplifies the code to review and is easy to understand. Using the artisan command, you can generate a request file.
php artisan make:request PostRequest
The above command will generate the PostRequest file inside the app\Http\Requests directory. So let’s add some validation rules inside the rules method of the PostRequest file.
public function rules()
{
return [
'title' => 'required|unique:posts|max:255',
'body' => 'required',
];
}
You need to type hint the request on your controller method. Before the controller method is called, it validates the incoming form request as below:
public function store(PostRequest $request)
{
// The incoming request is valid
// Retrieve the validated input data...
$validated = $request->validated();
}
If validation fails, it will generate a redirect response to send the user back to their previous location. If you want to add an “after” hook to the form request. You may use the withValidator() method. You may call any of its functions before they evaluate the validation rules.
public function withValidator($validator)
{
$validator->after(function ($validator) {
if ($this->somethingElseIsInvalid()) {
$validator->errors()->add('field', 'Something is wrong with this field!');
}
});
}
Authorizing Form Requests:
The Form request class presents the authorize() method. In that method, we are checking whether the user has the authority to update a resource.
public function authorize()
{
$comment = Comment::find($this->route('comment'));
return $comment && $this->user()->can('update', $comment);
}
If the authorize() method returns false, an HTTP response 403 status code will return. And the controller method will not execute. You have a plan to authorization logic in another part of your application. You should return true from the authorize() method.
Customizing the Error message:
You must override error messages in the Request class. This method contains an array of messages against fields.
public function messages()
{
return [
'title.required' => 'A title is required',
'body.required' => 'A message is required',
];
}
Customizing the validation attributes:
You would like to change the validation message to replace it with a custom attribute name. This method should return an array of attribute/name pairs.
public function attributes()
{
return [
'email' => 'email address',
];
}
Prepare Input for validation:
If you need to clean the data from the request before you apply validation rules, you can use the prepareForValidation() method:
protected function prepareForValidation()
{
$this->merge([
'slug' => Str::slug($this->slug),
]);
}
I am sure this article (how to validate user input with Laravel) helps you better understand the Form Request validation, displaying error messages in Laravel. I have followed this link for this article. If you have any doubts, then comment below then I will reply as per as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,