Your cart is currently empty!
How Learning Session In Laravel Could Save Your Money And Time.
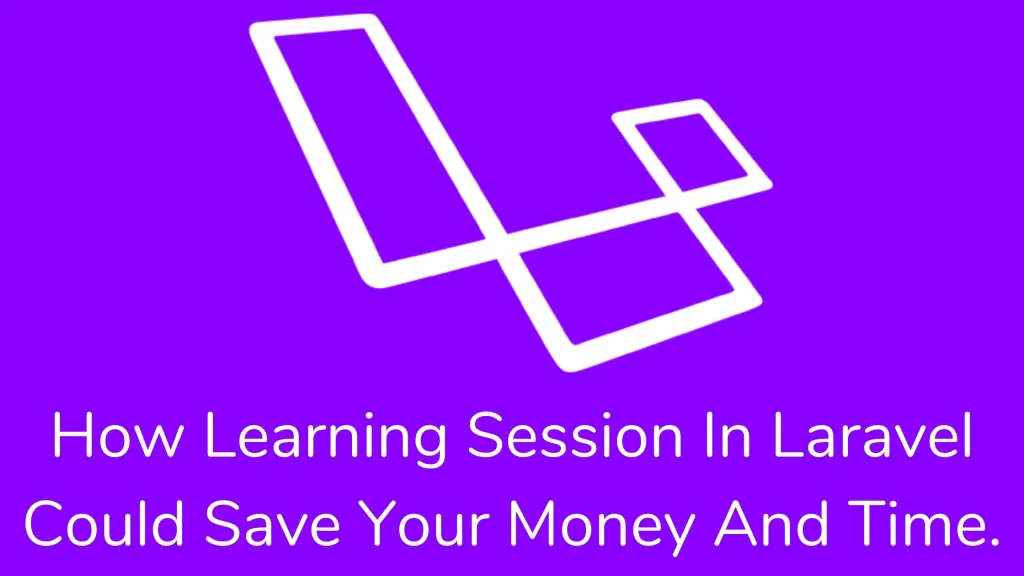
Hi guys,
Today we are learning how learning sessions in laravel could save you money and time. In this article, We are creating a custom Session driver for your laravel application. At the end of the article, you will get some information trick quiz. It will help you to increase your knowledge. So let’s begin.
Laravel provides you with a variety of session backends that are accessed through an expressive, unified API. The Session-related configuration is stored at session.php in the config folder. The driver option shows where the session file will be stored for each request. Laravel provides a variety of driver lists. The list of session drivers is given below:
- File – session stores in the storage/framework/session directory.
- Cookies – sessions are stored in secure, encrypted cookies.
- Database – sessions stored in a relational database.
- Memcached/Redis – sessions stored in one of these fast, cache-based stores.
- Array – sessions stored in a PHP array.
Driver Prerequisites:-
Database:
You want to store a session inside the database. You can easily create through the command in Laravel.
php artisan session:table
The above command to create a migration file that contains Schema to create a table structure like below:
Schema::create('sessions', function ($table) {
$table->string('id')->unique();
$table->unsignedInteger('user_id')->nullable();
$table->string('ip_address', 45)->nullable();
$table->text('user_agent')->nullable();
$table->text('payload');
$table->integer('last_activity');
});
Afterward, you must execute a migrate command. It will create a session table inside the database.
php artisan migrate
Using Session:-
Retrieving Data:
There are two ways to access session data in Laravel using the Request instance and session helper function.
First, We are going with the Request instance. It can be type-hinted on a controller method.
Note– Controller method dependencies are automatically injected via the service container.
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
class UserController extends Controller
{
/**
* Show the profile for the given user.
*
* @param Request $request
* @param int $id
* @return Response
*/
public function show(Request $request, $id)
{
$value = $request->session()->get('key');
//
}
}
You may pass the second argument as a default value to get the method. If the specified key does not exist, then it will return a default value. Also, you can pass a closure as a default value.
$value = $request->session()->get('key', 'default');
$value = $request->session()->get('key', function () {
return 'default';
});
Second, you can use the session PHP function to retrieve and store data in the session. Below are some examples that retrieve or store data in sessions.
Route::get('home', function () {
// Retrieve a piece of data from the session…
$value = session('key');
// Specifying a default value...
$value = session('key', 'default');
// Store a piece of data in the session...
session(['key' => 'value']);
});
If You would like to retrieve all session data, then you may use all() methods.
$data = $request->session()->all();
If you want to check, that an item is present in the session, then you may use the has() method. It returns true when the variable exists, and it is not null.
if ($request->session()->has('users')) {
//
}
The exists() method is used when you determine if an item is present in the session even though its value is null.
if ($request->session()->exists('users')) {
//
}
Storing data:
In the Request instance, the put() method to store data in the session.
$request->session()->put('key', 'value');
The push method is used to push a new value onto the session value If It is an array.
$request->session()->push('user.role', 'receptionist');
The pull method will retrieve and delete an item from the session.
$value = $request->session()->pull('key', 'default');
Flash Data:
Sometimes you may wish to store items in the session only for the next request. You may do so using the flash method. Flash data is primarily useful for short-lived status messages:
$request->session()->flash('status', 'Task was successful!');
You want to keep your flash data for several requests. You may use the reflash() method. If you only keep specific flash data, you may use the keep() method.
$request->session()->reflash();
$request->session()->keep(['username', 'email']);
The forget() method will remove a piece of data from the session. If you want to remove all session data, you may use the flush() method.
// Forget a single key…
$request->session()->forget('key');
// Forget multiple keys…
$request->session()->forget(['key1', 'key2']);
$request->session()->flush();
Regenerating the session ID:
Regenerating session ID prevents a malicious user from exploiting a session fixation attack on your application. Laravel automatically regenerates session ID during authentication if you are using a built-in LoginController. Also, you can renew the session ID manually.
$request->session()->regenrate();
Adding Custom Session Drivers:-
You would like to implement a custom session driver. You would implement SessionHandlerInterface like below:
<?php
namespace App\Extensions;
class MongoSessionHandler implements \SessionHandlerInterface
{
public function open($savePath, $sessionName) {}
public function close() {}
public function read($sessionId) {}
public function write($sessionId, $data) {}
public function destroy($sessionId) {}
public function gc($lifetime) {}
}
So a quick overview of the above methods and how they work-
- The open() method is used for the file-based session store system. But Laravel already uses a file session driver, So you need to keep it as it is.
- The close() method is used for the file-based session store system. For most drivers, it is not needed.
- The read() method should return the string version of session data associated with the given $sessionId.
- The write() method should write the given $data string associated with the $sessionId to some persistent storage system.
- The destroy() method should remove the data associated with the $sessionId from persistent storage.
- The gc() method will destroy all session data that is older than $lifetime.
Registering The Driver:
Once your driver has implemented it, you are ready to register it within the framework. You may extend the method on the Session facade inside the boot method of the service provider. You can use the existing AppServiceProvider or create a new Service Provider.
<?php
namespace App\Providers;
use App\Extensions\MongoSessionHandler;
use Illuminate\Support\Facades\Session;
use Illuminate\Support\ServiceProvider;
class SessionServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Session::extend('mongo', function ($app) {
// Return implementation of SessionHandlerInterface...
return new MongoSessionHandler;
});
}
}
Once you register the session driver in the Service provider, you can use it on your config/session.php configuration file.
I am pretty sure this article (How Learning Session In Laravel Could Save Your Money And Time) it help you a better understanding of the Session function in Laravel. I have followed this link for this article. If you have any doubts, then comment below then I will reply as per as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,