Your cart is currently empty!
How to integrate Firebase cloud messaging in Codeigniter
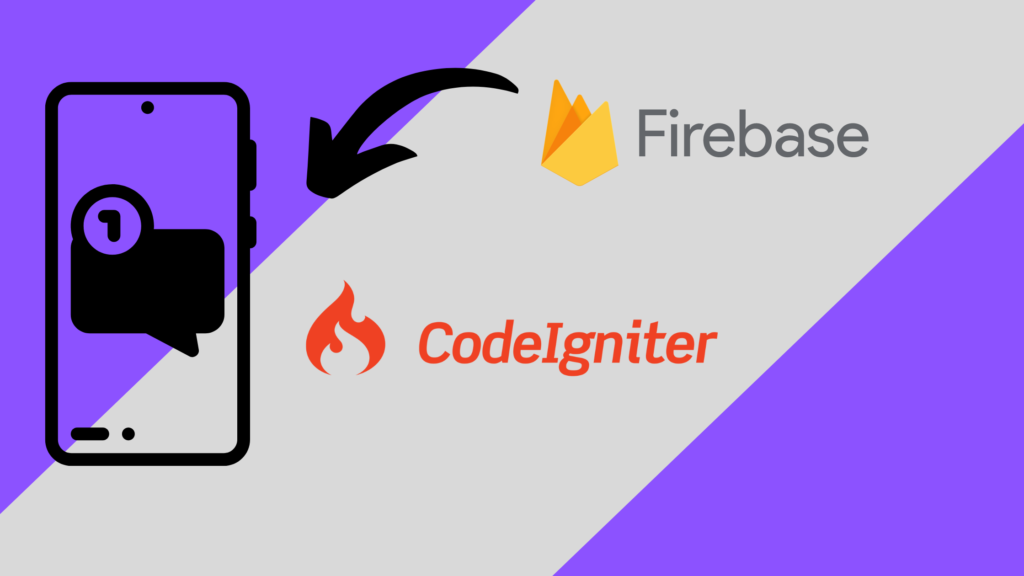
Hi guys,
Web notifications are messages sent to a visitor’s device from a website. It allows you to engage and retain website visitors. Push notifications can boost app engagement by 88%, with 66% of app users on average returning to that app when push is enabled.
We are working on CodeIgniter 3.* and Firebase Web notification. I am using Codeigniter-FCM-Library for the GitHub project. It is helpful to speed up the built time for the application. We will learn step by step:-
- Copy the androidfcm.php file from Codeigniter-FCM-Library and put it inside the config folder in your project.
<?php
defined('BASEPATH') or exit('No direct script access allowed');
/*
|||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|| Android Firebase Push Notification Configurations
|||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
*/
/*
|--------------------------------------------------------------------------
| Firebase API Key
|--------------------------------------------------------------------------
|
| The secret key for Firebase API
|
*/
$config['key'] = 'Enter your Server Key';
/*
|--------------------------------------------------------------------------
| Firebase Cloud Messaging API URL
|--------------------------------------------------------------------------
|
| The URL for Firebase Cloud Messaging
|
*/
$config['fcm_url'] = 'https://fcm.googleapis.com/fcm/send';
- Copy the fcm.php file from Codeigniter-FCM-Library and put it inside libraries in your project.
<?php
defined('BASEPATH') or exit('No direct script access allowed');
/**
* FCM simple server side implementation in PHP
*
* @author Abhishek
*/
class Fcm
{
/** @var string push message title */
private $title;
/** @var string message */
private $message;
/** @var string URL String */
private $image;
/** @var array Custom payload */
private $data;
/**
* flag indicating whether to show the push notification or not
* this flag will be useful when perform some opertation
* in background when push is recevied
*/
/** @var bool set background or not */
private $is_background;
/**
* Function to set the title
*
* @param string $title The title of the push message
*/
public function setTitle($title)
{
$this->title = $title;
}
/**
* Function to set the message
*
* @param string $message Message
*/
public function setMessage($message)
{
$this->message = $message;
}
/**
* Function to set the image (optional)
*
* @param string $imageUrl URI string of image
*/
public function setImage($imageUrl)
{
$this->image = $imageUrl;
}
/**
* Function to set the custom payload (optional)
*
* eg:
* $payload = array('user' => 'user1');
*
* @param array $data Custom data array
*/
public function setPayload($data)
{
$this->data = $data;
}
/**
* Function to specify if is set background (optional)
*
* @param bool $is_background
*/
public function setIsBackground($is_background)
{
$this->is_background = $is_background;
}
/**
* Generating the push message array
*
* @return array array of the push notification data to be send
*/
public function getPush()
{
$res = array();
$res['data']['title'] = $this->title;
$res['data']['is_background'] = $this->is_background;
$res['data']['message'] = $this->message;
$res['data']['image'] = $this->image;
$res['data']['payload'] = $this->data;
$res['data']['timestamp'] = date('Y-m-d G:i:s');
return $res;
}
/**
* Function to send notification to a single device
*
* @param string $to registration id of device (device token)
* @param array $message push notification array returned from getPush()
*
* @return array array of notification data and to address
*/
public function send($to, $message)
{
$fields = array(
'to' => $to,
'data' => $message,
);
return $this->sendPushNotification($fields);
}
/**
* Function to send notification to a topic by topic name
*
* @param string $to topic
* @param array $message push notification array returned from getPush()
*
* @return array array of notification data and to address (topic)
*/
public function sendToTopic($to, $message)
{
$fields = array(
'to' => '/topics/' . $to,
'data' => $message,
);
return $this->sendPushNotification($fields);
}
/**
* Function to send notification to multiple users by firebase registration ids
*
* @param array $to array of registration ids of devices (device tokens)
* @param array $message push notification array returned from getPush()
*
* @return array array of notification data and to addresses
*/
public function sendMultiple($registration_ids, $message)
{
$fields = array(
'registration_ids' => $registration_ids,
//'data' => $message,
'notification'=>$message,
);
return $this->sendPushNotification($fields);
}
/**
* Function makes curl request to firebase servers
*
* @param array $fields array of registration ids of devices (device tokens)
*
* @return string returns result from FCM server as json
*/
private function sendPushNotification($fields)
{
$CI = &get_instance();
$CI->load->config('androidfcm'); //loading of config file
// Set POST variables
$url = $CI->config->item('fcm_url');
$headers = array(
'Authorization: key=' . $CI->config->item('key'),
'Content-Type: application/json',
);
// Open connection
$ch = curl_init();
// Set the url, number of POST vars, POST data
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Disabling SSL Certificate support temporarly
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($fields));
// Execute post
$result = curl_exec($ch);
if ($result === false) {
die('Curl failed: ' . curl_error($ch));
}
// Close connection
curl_close($ch);
return $result;
}
}
- It’s better to create a helper function. So it is easy to call from any part of your application like below:
if(! function_exists('send_notification'))
{
function send_notification($userDevice,$message)
{
$CI = &get_instance();
$CI->load->library('fcm');
// $CI->fcm->setTitle('XXXXX');
// $CI->fcm->setMessage($message);
// $CI->fcm->setIsBackground(false);
// set payload as null
// $payload = array('notification' => '');
// $CI->fcm->setPayload($payload);
// $CI->fcm->setImage('https://firebase.google.com/_static/9f55fd91be/images/firebase/lockup.png');
// $json = $CI->fcm->getPush();
$notification = [
"title" => "XXXXX",
"body" => $message
];
/**
* Send to multiple
*
* @param array $token array of firebase registration ids (push tokens)
* @param array $json return data from getPush() method
*/
$result = $CI->fcm->sendMultiple($userDevice, $$notification);///$json
}
}
You can add the below line code on your application anywhere:
send_notification($userDevice,$message);
Creating App In Firebase Console:-
- Create a project on the Firebase Console Platform.
- Click to create a new Project.
- Add the Project Name and click the Continue button.
- I am enabling Google Analytics for a report.
- It will take less than 1 minute to create a project for you.
- Click Your Project Click the setting icon and click project settings.
- Goto Cloud messaging tab. You can copy the Server key and sender ID.
- We also want web push notifications. Click to create the keypair button.
- Goto General Tab, scroll down a little. We need to create a web app.
- Enter the web app name and click to register.
I am using a <script> tag. Copy the code from the screen. You can add this code on a common file that loads all the time like footer.php inside the views folder. You will need to some changes. So, the User’s browser will alert for notification permission. After the user grants the permission. The user will get a notification when the admin updates information.
<script src="https://www.gstatic.com/firebasejs/5.7.2/firebase.js"></script>
<script src="https://www.gstatic.com/firebasejs/5.7.0/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/5.7.0/firebase-messaging.js"></script>
<script>
const firebaseConfig = {
apiKey: "API Key",
authDomain: "XXXXXXXX.firebaseapp.com",
projectId: "XXXXXXXX",
storageBucket: "XXXXXXXX.appspot.com",
messagingSenderId: "AAAAAAAAAAA",
appId: "XXXXXXXXXXX",
measurementId: "G-XXXXXXXX"
};
// Initialize Firebase
firebase.initializeApp(firebaseConfig);
const messaging = firebase.messaging();
messaging.usePublicVapidKey('KEYPAIR KEY');
messaging.requestPermission().then(function(){
console.log('Notification permission granted')
messaging.getToken().then(function(currentToken){
console.log(currentToken)
}).catch(function(err){
console.log('error occur ', err);
showToken('token id ',err);
setTokenSentToServer(false);
})
}).catch(function(err){
console.log('Unable get permission from user ',err);
})
messaging.onMessage(function(payload){
var obj = payload.notification ///JSON.parse(payload.notification)
var notification = new Notification(obj.title,{
icon: obj.icon,
body: obj.body
})
})
</script>
Note:- I have removed the testfcm project from my Firebase console. You can add your project credentials to the CodeIgniter application.
If your application of PWA, you need to create the file “firebase-messaging-sw.js” inside the root directory. Add the below code to the file.
importScripts('https://www.gstatic.com/firebasejs/9.6.1/firebase-app.js');
importScripts('https://www.gstatic.com/firebasejs/9.6.1/firebase-messaging.js');
firebase.initializeApp({
'messageSenderId':'208000316551',
});
const messaging = firebase.messaging();
messaging.setBackgroundMessageHandler(function(payload){
console.log('received background message ',payload);
var obj = JSON.parse(payload.data.notification)
var title = obj.title;
var options = {
body: obj.body,
icon: obj.icon
};
return self.registration.showNotification(title, options);
});
You need to add the below code
async function registerSW() {
if ('serviceWorker' in navigator) {
try {
//////
await navigator.serviceWorker.register("/firebase-messaging-sw.js");
}
catch (e) {
console.log('SW registration failed');
}
}
}
I hope that this post (How to integrate Firebase Cloud messaging in Codeigniter) has helped you understand integrate Firebase Cloud messaging into Codeigniter. Please leave a remark if you have any queries, and I will answer as quickly as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,