Your cart is currently empty!
Dig deep into the architectural concept of laravel
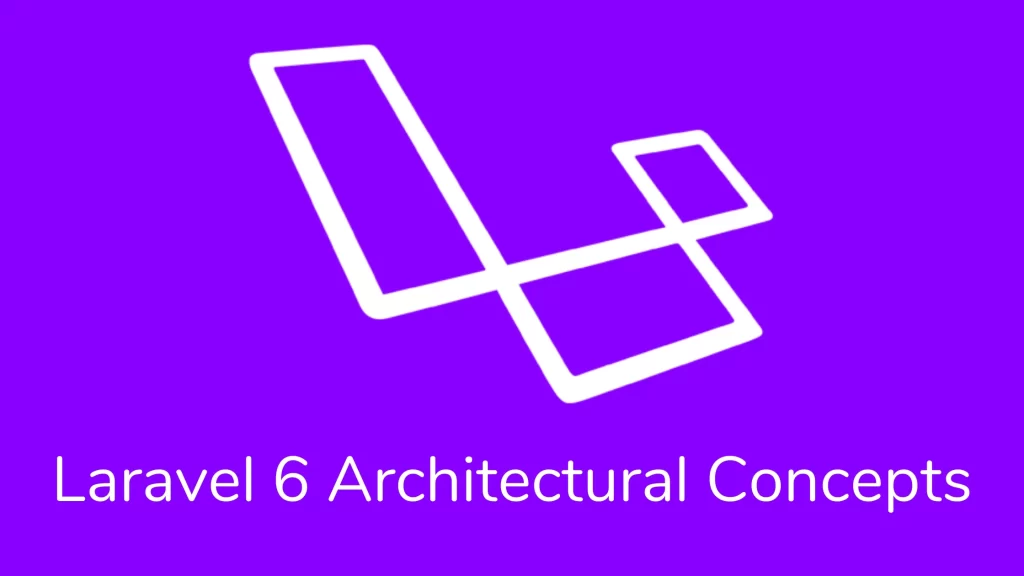
Hi guys,
In this article, we are going to look at architectural behavior in laravel. Laravel framework is one of the best frameworks. Architectural has four topics given below:
Check given topics of Architectural Concept of Laravel
Request Lifecycle:-
The entry point for all requests to the Laravel Application is the index.php file inside a public folder. The index.php file contains less amount of code, which loads the composer autoloader and retrieves an instance of laravel.
HTTP kernel:
All Request goes through HTTP Kernel or Console Kernel. The HTTP kernel and Console kernel act as the central point of the application. Before the Request is processed, Kernel handles it via Middleware. HTTP Kernel contains a handle method that receives Requests and returns responses.
Service Provider:
It is a significant aspect of the Laravel bootstrap process. The service provider is responsible for bootstrapping all various components. The Configuration file (config/app.php) contains all service provider lists as an array. All service providers have registered when a boot method is called. The Request is handed over to the router for further process. Laravel’s core service and your application are bootstraps through a service provider. The service providers are the central point to configure your application. Using the below command, you can make a new service provider. In the Register method, write no events listeners, routes, or any functionality.
php artisan make:provider TestServiceProvider
Service Container:-
We have used the service container to create big-scale applications and build a power application. It is the middle layer between the request and the database. In a small project, we access the database directly, which is good. But in large applications, it is more complicated, so in that case, a Service container takes place.
Service Container is bound when application bootstrap applications are within service providers. There is no need to bind classes into the container if they do not depend on any interfaces. The binding syntax is given below:
$this->app->bind('Repository Interface path ','Repository Class path');
for example,
$this->app->bind('app\Repositories\UserRepositoryInterface','app\Repositories\UserRepository');
I have added this line inside the AppServiceProvider.php on the boot method.
Also, you can use a singleton for binding a class or an interface. The singleton will resolve at one time, and the same instance is a return for all subsequent calls. The syntax for the singleton is below:
$this->app->singleton('Repository Interface path','Repository class path');
Sometimes you need an injected primitive value such as an integer. So you use the injected value in your class. For example,
$this->app->when('App\Http\Controllers\UserController')
->needs('$variableName')
->give($value);
Facades:-
Facades provide catchy syntax that allows you to use Laravel features. A Facade is a class that provides access to an object from the container.
Contracts:-
Set of interfaces that define core service provided by the framework. If you are building an external package, then recommended to use a contract.
<?php
namespace App\Orders;
class Repository
{
/**
* The cache instance.
*/
protected $cache;
/**
* Create a new repository instance.
*
* @param \SomePackage\Cache\Memcached $cache
* @return void
*/
public function __construct(\SomePackage\Cache\Memcached $cache)
{
$this->cache = $cache;
}
/**
* Retrieve an Order by ID.
*
* @param int $id
* @return Order
*/
public function find($id)
{
if ($this->cache->has($id)) {
//
}
}
}
The above code is tightly coupled because we depend on the cache class from a third-party package.
Instead of this approach, we can improve our code by depending on a simple vendor-agnostic interface:
<?php
namespace App\Orders;
use Illuminate\Contracts\Cache\Repository as Cache;
class Repository
{
/**
* The cache instance.
*/
protected $cache;
/**
* Create a new repository instance.
*
* @param Cache $cache
* @return void
*/
public function __construct(Cache $cache)
{
$this->cache = $cache;
}
}
The above code does not depend on the third-party package. If we want to change the technology, then we should change the contract cache implementation.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,