Your cart is currently empty!
How to Upload Images in Laravel Application
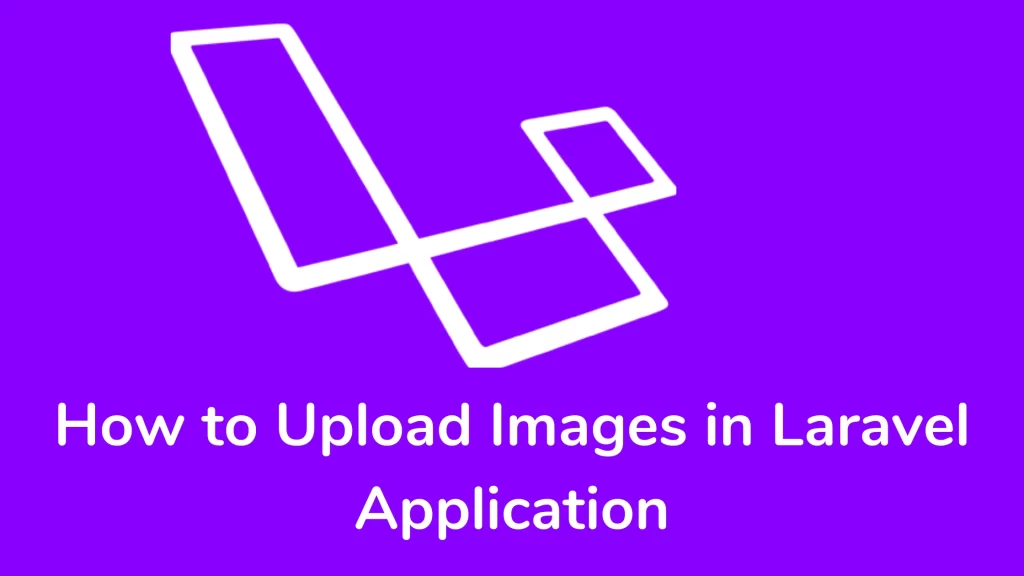
Hi,
In this article, we can upload files in laravel applications like PDF, doc, txt, jpeg, and png, We will upload images in laravel. You can make small changes in another extension. It works well.
Follow the below steps to upload images in the Laravel application.
Install Laravel Framework
If you have not made a laravel application, you can run the below command or skip this step.
composer require laravel/laravel image_uploader
Make View for upload image
We have to create a form to upload the image. We will make an uploadFile.blade.php file. Check the below code, and you can update your code accordingly.
<div class='container'>
@if(Session::get('message'))
<div class='alert {{$alertClass}}'>
<button type='button' class='close' data-dismiss='alert'>x</button>
<b>{{$message}}</b>
</div>
@endif
<form action='{{route("image.upload")}}' enctype='multipart/form-data' method='post'>
<div class='form-group'>
<input type='file' name='image' class='form-control @error("image") is-valid @enderror'>
@error('image')
<span class='text-danger'>{{$message}}</span>
@enderror
</div>
<div class='mt-3'>
<button type='submit' class='btn btn-success'>Upload</button>
</div>
</form>
</div>
Add Routes on the web
We need to add routes in the web.php file. For this tutorial, we have added GET and POST routes. Check the below routes list.
use AppHttpControllersUploadController;
Route::get('upload-file',[UploadController::class, 'create']);
Route::post('upload-file',[UploadController::class, 'store']);
Create a Controller
For upload, we need to make a controller using the below command.
php artisan make:controller UploadController
In the code below, we store the image in the ‘image’ folder inside the public directory.
<?php
namespace AppHttpControllers;
use IlluminateHttpRequest;
class UploadController extends Controller
{
public function create()
{
return view('upload.create');
}
public function store(Request $request)
{
$request->validate([
'image' => 'required|image|mimes:jpeg,png,jpg|max:1024',
]);
$name = time().'-'.$request->image->extension();
$request->image->move(public_path('images'), $name);
return back()->with(['success'=>'successfully uploaded image','class'=>'alert-success']);
}
}
You can also use the below code for a store inside the storage folder.
$request->image->storeAs('images', $name);
// storage/app/images/file.png
If you store the file on the Amazon S3 server, we can use the below code to upload the image file.
$request->image->storeAs('images', $image, 's3');
We have successfully uploaded images to the laravel application. You can also upload images on the Amazon S3 bucket.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers