Your cart is currently empty!
What is hashing in laravel & learn its algorithm.
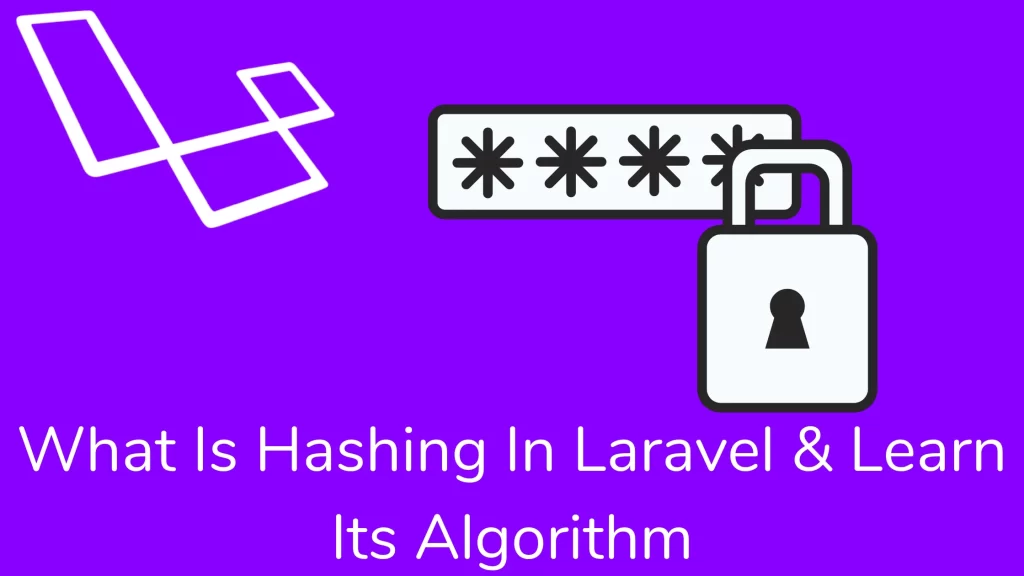
Hi guys,
We’ll look into What Is Hashing In Laravel & Learn Its Algorithm. The technique of converting any given key or string of characters into another value is known as hashing. It is recommended that the user credentials be hashed.
Laravel uses Becrypt and Aragon2 hashing algorithms to store user passwords using hash Facade. You are using built-in LoginController and RegisterController classes in your application. It will use bcrypt hash for registration and authentication.
Configuration:-
You can change the hashing driver inside the config/hashing.php file in your application. It supports three drivers: bcrypt, argon2i, and argon2id.
Basic Usage:-
You may hash a password using the make method from the Hash facade:
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
class UpdatePasswordController extends Controller
{
/**
* Update the password for the user.
*
* @param Request $request
* @return Response
*/
public function update(Request $request)
{
// Validate the new password length
$request->user()->fill([
'password' => Hash::make($request->newPassword)
])->save();
}
}
The make() method:
The make method allows you to manage the work factor of the algorithm using the rounds option for the bcrypt algorithm. It will control how many iterations the make method will use when calculating the final hash.
$hashed = Hash::make('password', [
'rounds' => 12,
]);
If you are using the argon2 algorithm, you can pass memory, time, and threads to make a method for managing the work factor. The memory option is memory allocated to make hashing. The time option is how many iterations make methods to generate a final hash. They use the thread option to how many threads work to make a hash.
$hashed = Hash::make('password', [
'memory' => 1024,
'time' => 2,
'threads' => 2,
]);
check() method:
The check method allows you to verify plain text string and given hashed string. If you are using the default LoginController Controller, then you will not need to use this directly.
if (Hash::check('plain-text', $hashedPassword)) {
// The passwords match…
}
The needsRehash() method:
The needsRehash function allows you to determine if the work factor used by the hasher has changed since the password hashed.
if (Hash::needsRehash($hashed)) {
$hashed = Hash::make('plain-text');
}
I hope that this post (What Is Hashing In Laravel & Learn Its Algorithm) has helped you understand how to make, and check the hash from Hash Facade, and also you regenerate hash string. I used this site to write this post. Please leave a remark if you have any queries, and I will answer as quickly as possible.
Thank you for reading this article. Please share this article. That’s it for the day. Stay Connected!
Cheers,