Your cart is currently empty!
A simple way to learn how to compiled assets in laravel
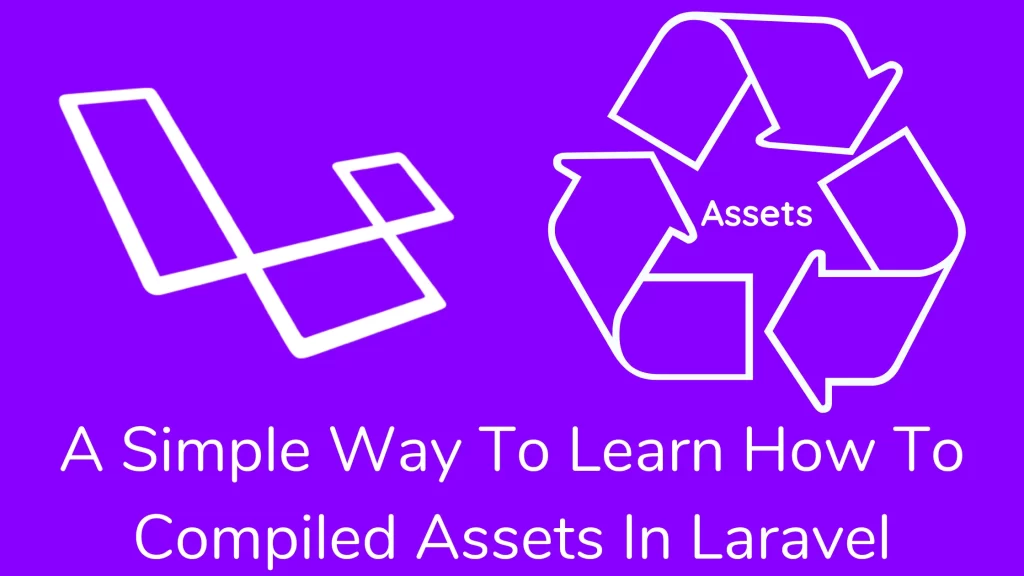
Hi guys,
We’ll take a look at Laravel’s compiled assets. In this article. We’ll use the Laravel mix package to compile the JS and CSS files. We’ll learn how to use less, sass, stylus, and PostCSS to create compiled assets in laravel, as well as how to use environment variables as mix variables. Continue reading to gain the most up-to-date information about asset compilation.
Laravel Mix provides a fluent API for Webpack build steps for your laravel application using any CSS and JS Preprocessors.
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css');
Before building your application, Make sure that node.js and NPM are on your system. By default Laravel, it includes some packages in the package.json file for a kick start. Run the following command that will install all dependencies for your application.
npm install
The package.json file contains scripts that execute Mix tasks. Below, command compiled assets of your project.
npm run dev
npm run production //to build production-ready code
npm run watch // watch all relevant files for changes.
Working with Stylesheet:-
Mix tasks can be chained together to define correctly. How your assets to compiled.
Less:
The less method compiles and converts from less into CSS. You can write the below code in the webpack.mix.js file.
mix.less('resources/less/app.less','public/css');
Also, you can compile multiple files through the less() method.
mix.less('resources/less/app.less','public/css')
.less('resources/less/custom.less','public/css');
You can also change output file names. It will rename to the output file.
mix.less('resources/less/app.less', 'public/stylesheets/styles.css');
Sass:
The Sass method will convert files from Sass to CSS. You can write it as below:
mix.sass('resources/sass/app.sass','public/css');
You can also pass multiple files to the sass() method like below.
mix.sass('resources/sass/app.sass', 'public/css')
.sass('resources/sass/admin.sass', 'public/css/admin');
Stylus:
You can also convert stylus files into CSS using the stylus() method.
mix.stylus('resources/stylus/app.stylus','public/css');
PostCSS:
it is a powerful tool for transforming your CSS. you can install any plugin for your application.
mix.sass('resources/sass/app.scss', 'public/css')
.options({
postCss: require('postcss-css-variables')()
});
Plain CSS:
you concatenate all CSS files into a single file using the styles method:
mix.styles([
'public/css/vendor/normalize.css',
'public/css/vendor/videojs.css'
], 'public/css/all.css');
URL Processing:
Laravel Mix is built on top of Webpack. Webpack will rewrite and optimize all URL calls within your style sheets.
.example {
background: url('../images/example.png');
}
If you want to disable the rewritten URL. add options() method to it like below:
mix.sass('resources/sass/app.scss', 'public/css')
.options({
processCssUrls: false
});
Source Maps:
A source map is disabled by default in laravel. You can activate it by defining the mix.sourceMaps() method in your webpack.mix.js file. Webpack offers you different mapping styles.
mix.js('resources/js/app.js', 'public/js')
.sourceMaps();
let productionSourceMaps = false;
mix.js('resources/js/app.js', 'public/js')
.sourceMaps(productionSourceMaps, 'source-map');
Working with Javascript:-
A single update to update the application code will force the browser to re-download all your vendor libraries even if not changed. The Mix method accepts an array of libraries or modules that you wish to extract into a vendor.js file.
mix.js('resources/js/app.js', 'public/js')
.extract(['vue'])
Load these files to avoid errors.
/// The Webpack manifest runtime
<script src="/js/manifest.js"></script>
/// Your vendor libraries
<script src="/js/vendor.js"></script>
/// Your application code
<script src="/js/app.js"></script>
React:
You want to make your application in React. You need to replace mix.js() to mix.react(). it will automatically download Babel plugins for your application.
mix.react('resources/js/app.jsx', 'public/js');
Vanilla Js:
You can combine and minify many javascript files with the scripts() method.
mix.scripts([
'public/js/admin.js',
'public/js/dashboard.js'
], 'public/js/all.js');
Custom Webpack Configuration:-
You want to customize your webpack.config.js
There are two ways to configure.
Merging Custom Configuration:
The webpackConfig() method allows adding Webpack configuration for overrides.
mix.webpackConfig({
resolve: {
modules: [
path.resolve(__dirname, 'vendor/laravel/spark/resources/assets/js')
]
}
});
Custom Configuration Files:
You can copy a file from node_modules/laravel-mix/setup/webconfig.config.js file to the parent’s root directory. Afterward, point all config files in the –config reference package.json file.
Copying Files & Directories:-
The copy() method may copy files and directories to new locations. You can also use the copyDirectory() method. To copy all directory structures to the destination.
mix.copy('node_modules/foo/bar.css', 'public/css/bar.css');
mix.copyDirectory('resources/img', 'public/img');
It is useful when node_modules contain particular assets. You require it for your application.
Versioning/ Cache Busting:-
The Version method will automatically append a hash to the compiled file name. So that forces browsers to load new assets instead of serving copied code.
mix.js('resources/js/app.js', 'public/js')
.version();
You do not know what is the file name after compiling scripts. So, you use the mix function to load compiled scripts. It will automatically determine the current hashed file name.
Your compiled assets deploy to a CDN separate from your application. You must change mix_url on the config/app.php file.
'mix_url' => env('MIX_ASSET_URL', null)
Browsersync Reloading:-
Browsersync is checking your files for changes. It will reflect changes in the browser without manual reloading.
mix.browserSync('project.test.com')
You are changing scripts or PHP files for your application. The watch command refreshes the page to reflect your changes.
Environment Variables:-
You may inject environment variables into the associate by adding the MIX_ key to variables.
MIX_SENTRY_DSN_PUBLIC=http://example.com
After defining the variable inside your .env file, it may access it via the process.env object.
process.env.MIX_SENTRY_DSN_PUBLIC
Notifications:-
After compiling project assets, the mix will automatically notify the user whether it compiles successfully or not. You disable that notification using the disableNotifications() method:
mix.disableNotifications();
I hope this article (Easy way to Learn how to compiled assets in Laravel) helps you better understand compiling your assets so it speeds up your production release. For this article, I followed this link. If you have questions, please leave a comment and I will respond as soon as possible.
Thank you for reading this article. Please share this article with your friend circle. That’s it for the day. Stay Connected!
Cheers,